已知class ControlArc { public: std::string taskId; std::string satelliteName; std::string satelliteId; std::chrono::system_clock::time startTime; std::chrono::system_clock::time endTime; long long dt};std::vector<std::vector> ControlArcList , ControlArcList.size=4, 任意一个std::vector 中的startTime且endTime时间段都有可能和其余3个std::vector中的startTime且endTime时间段有重叠,请给出c++代码使得任意一个std::vector 中的startTime且endTime时间段都不和其余3个std::vector中的startTime且endTime时间段有重叠,有重重叠的筛选规则是ControlArc 中dt值大的
时间: 2023-07-08 13:30:28 浏览: 80
以下是一种可能的实现方式:
```c++
#include <vector>
#include <algorithm>
bool overlap(const ControlArc& arc1, const ControlArc& arc2) {
return arc1.endTime > arc2.startTime && arc2.endTime > arc1.startTime;
}
bool compare(const ControlArc& arc1, const ControlArc& arc2) {
return arc1.dt > arc2.dt;
}
void filterControlArcList(std::vector<std::vector<ControlArc>>& ControlArcList) {
for (auto& arcList : ControlArcList) {
std::sort(arcList.begin(), arcList.end(), compare);
for (size_t i = 0; i < arcList.size(); ++i) {
bool overlapFlag = false;
for (auto& otherList : ControlArcList) {
if (&otherList == &arcList) continue;
for (auto& otherArc : otherList) {
if (overlap(arcList[i], otherArc)) {
overlapFlag = true;
break;
}
}
if (overlapFlag) break;
}
if (overlapFlag) {
arcList.erase(arcList.begin() + i);
--i;
}
}
}
}
```
首先定义了一个 `overlap` 函数,用于判断两个 `ControlArc` 对象的时间段是否有重叠。然后定义了一个 `compare` 函数,用于在排序时按照 `ControlArc` 对象的 `dt` 值从大到小排序。最后定义了一个 `filterControlArcList` 函数,用于过滤 `ControlArc` 对象列表。具体实现如下:
- 对于每个 `ControlArc` 对象列表,首先按照 `dt` 值从大到小排序,以确保在有重叠的情况下优先保留 `dt` 值大的对象;
- 然后遍历每个 `ControlArc` 对象,检查它是否与其他列表中的对象重叠;
- 如果有重叠,则从当前列表中删除该对象。
这样,最终每个 `std::vector` 中的 `ControlArc` 对象的时间段都不会与其他 `std::vector` 中的对象重叠,并且在有重叠的情况下会保留 `dt` 值大的对象。
相关推荐
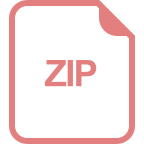
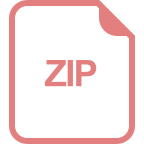
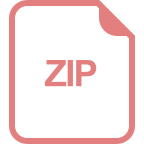















