opencv c++通过傅里叶变换去除正弦噪声
时间: 2023-09-10 08:10:45 浏览: 43
傅里叶变换可以将信号从时域转换到频域,因此可以用于去除正弦噪声。以下是使用OpenCV C++实现去除正弦噪声的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <cmath>
using namespace std;
using namespace cv;
int main()
{
Mat img = imread("lena.jpg", IMREAD_GRAYSCALE);
if (img.empty())
{
cout << "Failed to read image!" << endl;
return -1;
}
// Add sinusoidal noise
int amplitude = 30;
double frequency = 0.05;
for (int i = 0; i < img.rows; i++)
{
for (int j = 0; j < img.cols; j++)
{
int value = img.at<uchar>(i, j) + amplitude * sin(2 * CV_PI * frequency * j);
img.at<uchar>(i, j) = saturate_cast<uchar>(value);
}
}
// Perform Fourier transform
Mat imgFreq;
dft(img, imgFreq, DFT_COMPLEX_OUTPUT);
// Shift the zero-frequency component to the center of the spectrum
Mat imgFreqShifted;
imgFreqShifted = imgFreq.clone();
int cx = imgFreqShifted.cols / 2;
int cy = imgFreqShifted.rows / 2;
Mat q0(imgFreqShifted, Rect(0, 0, cx, cy));
Mat q1(imgFreqShifted, Rect(cx, 0, cx, cy));
Mat q2(imgFreqShifted, Rect(0, cy, cx, cy));
Mat q3(imgFreqShifted, Rect(cx, cy, cx, cy));
Mat tmp;
q0.copyTo(tmp);
q3.copyTo(q0);
tmp.copyTo(q3);
q1.copyTo(tmp);
q2.copyTo(q1);
tmp.copyTo(q2);
// Create a mask to filter out the sinusoidal noise
Mat mask(img.size(), CV_32FC1, Scalar(0));
int radius = 20;
Point center(img.cols / 2, img.rows / 2);
circle(mask, center, radius, Scalar(1), -1);
// Apply the mask to the frequency spectrum
Mat imgFreqFiltered;
multiply(imgFreqShifted, mask, imgFreqFiltered);
// Perform inverse Fourier transform
Mat imgFiltered;
idft(imgFreqFiltered, imgFiltered, DFT_SCALE | DFT_REAL_OUTPUT);
// Show the results
namedWindow("Original", WINDOW_AUTOSIZE);
imshow("Original", img);
namedWindow("Noisy", WINDOW_AUTOSIZE);
imshow("Noisy", imgFreqShifted);
namedWindow("Filtered", WINDOW_AUTOSIZE);
imshow("Filtered", imgFiltered);
waitKey(0);
return 0;
}
```
该代码首先将lena图像加载为灰度图像,然后添加正弦噪声。接下来,它执行傅里叶变换,并将零频率分量移动到频谱的中心。然后,它创建一个掩膜,以过滤掉正弦噪声。通过将掩膜应用于频谱,可以得到过滤后的频谱。最后,它执行逆傅里叶变换以恢复图像,并显示原始图像、噪声图像和过滤后的图像。
相关推荐
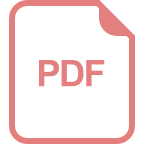
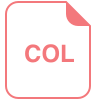
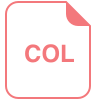
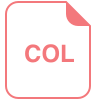
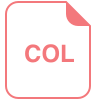
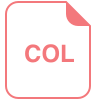




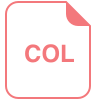
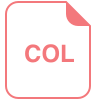
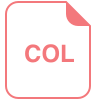