java将geojson转换为图片详细代码
时间: 2024-02-26 09:54:03 浏览: 358
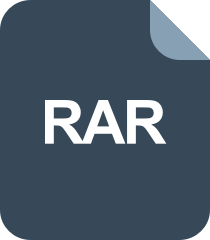
java处理图片代码

以下是使用Java将GeoJSON转换为图片的详细代码:
```java
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.geom.Rectangle2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileInputStream;
import java.util.List;
import javax.imageio.ImageIO;
import org.geojson.Feature;
import org.geojson.FeatureCollection;
import org.geojson.GeoJsonObject;
import org.geojson.LngLatAlt;
import org.geojson.Point;
import org.geojson.Polygon;
import org.geojson.jackson.JsonParser;
import org.geojson.jackson.ObjectMapper;
public class GeoJsonToImage {
public static void main(String[] args) throws Exception {
// 读取GeoJSON文件
FileInputStream fis = new FileInputStream(new File("input.geojson"));
ObjectMapper mapper = new ObjectMapper();
JsonParser parser = new JsonParser(mapper);
FeatureCollection featureCollection = parser.parseFeatureCollection(fis);
// 计算GeoJSON数据的范围
double minX = Double.POSITIVE_INFINITY;
double minY = Double.POSITIVE_INFINITY;
double maxX = Double.NEGATIVE_INFINITY;
double maxY = Double.NEGATIVE_INFINITY;
for (Feature feature : featureCollection.getFeatures()) {
GeoJsonObject geometry = feature.getGeometry();
if (geometry instanceof Point) {
LngLatAlt coordinates = ((Point) geometry).getCoordinates();
minX = Math.min(minX, coordinates.getLongitude());
minY = Math.min(minY, coordinates.getLatitude());
maxX = Math.max(maxX, coordinates.getLongitude());
maxY = Math.max(maxY, coordinates.getLatitude());
} else if (geometry instanceof Polygon) {
List<List<LngLatAlt>> coordinates = ((Polygon) geometry).getCoordinates();
for (List<LngLatAlt> ring : coordinates) {
for (LngLatAlt point : ring) {
minX = Math.min(minX, point.getLongitude());
minY = Math.min(minY, point.getLatitude());
maxX = Math.max(maxX, point.getLongitude());
maxY = Math.max(maxY, point.getLatitude());
}
}
}
}
// 计算图片的大小和比例
int width = 800;
int height = (int) (800 * (maxY - minY) / (maxX - minX));
double xRatio = width / (maxX - minX);
double yRatio = height / (maxY - minY);
// 创建图片
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = image.createGraphics();
g2d.setBackground(Color.WHITE);
g2d.clearRect(0, 0, width, height);
// 绘制GeoJSON数据
for (Feature feature : featureCollection.getFeatures()) {
GeoJsonObject geometry = feature.getGeometry();
if (geometry instanceof Point) {
LngLatAlt coordinates = ((Point) geometry).getCoordinates();
int x = (int) ((coordinates.getLongitude() - minX) * xRatio);
int y = (int) ((maxY - coordinates.getLatitude()) * yRatio);
g2d.setColor(Color.RED);
g2d.fillRect(x - 5, y - 5, 10, 10);
} else if (geometry instanceof Polygon) {
List<List<LngLatAlt>> coordinates = ((Polygon) geometry).getCoordinates();
for (List<LngLatAlt> ring : coordinates) {
int[] xPoints = new int[ring.size()];
int[] yPoints = new int[ring.size()];
for (int i = 0; i < ring.size(); i++) {
LngLatAlt point = ring.get(i);
int x = (int) ((point.getLongitude() - minX) * xRatio);
int y = (int) ((maxY - point.getLatitude()) * yRatio);
xPoints[i] = x;
yPoints[i] = y;
}
g2d.setColor(Color.GREEN);
g2d.fillPolygon(xPoints, yPoints, ring.size());
}
}
}
// 保存图片
ImageIO.write(image, "png", new File("output.png"));
// 关闭流
fis.close();
}
}
```
这个程序使用 `org.geojson` 包解析 GeoJSON 数据,绘制点和多边形,并保存为 PNG 图片。你可以替换 `org.geojson` 包为其它 GeoJSON 解析库,以适应你的需求。
阅读全文
相关推荐
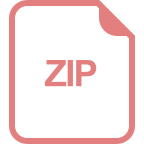
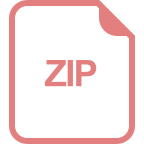


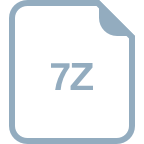
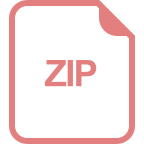
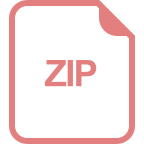
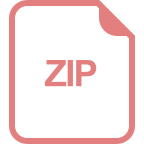
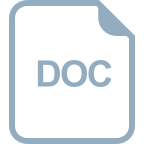








