TCN实现一维单特征时间序列信号端到端分类代码,有100个样本,每个样本数据是一个2000长的数据,每个数据只有一个数,希望输出2000个0-6七分类的标签,每类之间的变化位置不明确需要模型去学习,pytorch实现
时间: 2024-03-27 12:35:05 浏览: 12
好的,以下是基于PyTorch的TCN模型的代码示例,用于实现一维单特征时间序列信号的端到端分类。代码注释中会有一些解释,希望对您有所帮助。
```python
import torch
import torch.nn as nn
# 定义TCN模型
class TCN(nn.Module):
def __init__(self, input_size, output_size, num_channels, kernel_size=2, dropout=0.2):
super(TCN, self).__init__()
self.input_size = input_size
self.output_size = output_size
self.num_channels = num_channels
self.kernel_size = kernel_size
self.dropout = dropout
self.layers = self._create_layers()
def _create_layers(self):
layers = []
num_levels = len(self.num_channels)
# 创建卷积层
for i in range(num_levels):
dilation_size = 2 ** i
in_channels = self.input_size if i == 0 else self.num_channels[i-1]
out_channels = self.num_channels[i]
layers += [nn.Conv1d(in_channels, out_channels, self.kernel_size, dilation=dilation_size),
nn.BatchNorm1d(out_channels),
nn.ReLU(),
nn.Dropout(p=self.dropout)]
# 创建全局池化层
layers += [nn.AdaptiveMaxPool1d(1)]
# 创建输出层
layers += [nn.Linear(self.num_channels[-1], self.output_size)]
return nn.Sequential(*layers)
def forward(self, x):
return self.layers(x)
# 定义输入数据形状
input_size = 1
sequence_length = 2000
output_size = 7
num_channels = [64, 128, 256]
# 创建TCN模型
model = TCN(input_size, output_size, num_channels)
# 打印模型概况
print(model)
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=1e-3)
# 生成虚拟数据
x_train = torch.randn(100, input_size, sequence_length)
y_train = torch.randint(output_size, size=(100, sequence_length))
# 训练模型
for epoch in range(100):
# 将输入数据转换为PyTorch张量
x_train_tensor = torch.tensor(x_train, dtype=torch.float32)
y_train_tensor = torch.tensor(y_train, dtype=torch.long)
# 前向传播
y_pred = model(x_train_tensor)
# 计算损失
loss = criterion(y_pred.view(-1, output_size), y_train_tensor.view(-1))
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印损失
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, 100, loss.item()))
# 生成虚拟测试数据
x_test = torch.randn(1, input_size, sequence_length)
# 进行预测
with torch.no_grad():
y_pred = model(x_test)
# 打印预测结果
print("y_pred shape:", y_pred.shape)
```
希望这个基于PyTorch的TCN模型的代码示例能够对您有所帮助。
相关推荐
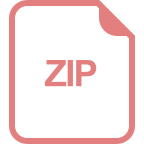
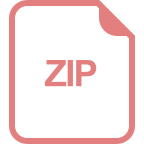
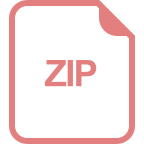





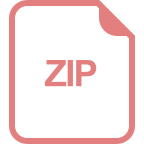
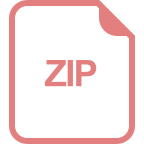
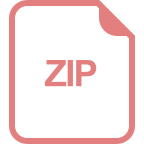
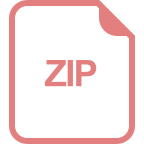
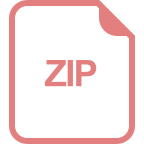
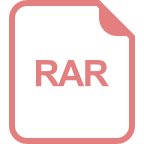
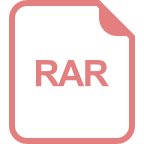
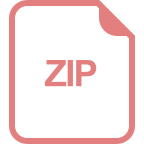
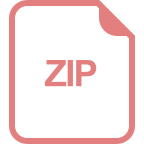