sum=0 for i in range(0,10):(0,1,2,3,4,5,6,7,8,9) if i%2==0: sum-=i else: sum+=i print(sum)
时间: 2024-05-16 09:17:57 浏览: 157
这段代码的作用是计算 0 到 9 中所有奇数的和,再减去所有偶数的和,最后输出结果。
具体来说,代码中使用了一个 for 循环,遍历了 0 到 9 的所有数字。对于每个数字 i,如果它是偶数,则将 sum 减去 i,否则将 sum 加上 i。最后输出 sum 的值。
可以将代码进行逐行解释:
```python
sum = 0 # 初始化 sum 为 0
for i in range(0, 10): # 遍历 0 到 9 的所有数字
if i % 2 == 0: # 如果 i 是偶数
sum -= i # 将 sum 减去 i
else: # 否则,也就是 i 是奇数
sum += i # 将 sum 加上 i
print(sum) # 输出 sum 的值
```
因为 0 和偶数的和为偶数,而奇数的和为奇数,所以最终的结果就是所有奇数的和减去所有偶数的和。在这个例子中,最终的结果为 5。
相关问题
sum = 0 for i in range(10): if i // 3 == 2: continue sum = sum + i print(sum)
这段代码是有语法错误的,应该是:
```python
sum = 0
for i in range(10):
if i // 3 == 2:
continue
sum += i
print(sum)
```
这段代码的作用是计算在 0 到 9 的范围内,除以 3 的商等于 2 的数除外,其余数的和。因为当 i // 3 == 2 时,使用 continue 跳过了该循环,所以不会将 i 的值加到 sum 中。最终输出的是符合条件的数的和,即 0 + 1 + 2 + 4 + 5 + 7 + 8 = 27。
sum = 0 for i in range(1, 11): sum += i print(sum)
This code will output the value of the variable "sum" after each iteration of the for loop.
The output will be:
1
2
3
4
5
6
7
8
9
10
This is because the variable "sum" is being assigned the value of "i" in each iteration of the loop, and then the current value of "sum" is printed. Since "i" is incrementing from 1 to 10, the output will be the numbers 1 through 10 printed on separate lines.
Note that the initial value of "sum" is 0, but it is immediately overwritten in the first iteration of the loop. If the intention was to add up the values of "i" from 1 to 10, the code should be modified as follows:
sum = 0
for i in range(1, 11):
sum += i
print(sum)
This would output the value of the variable "sum" after the loop has completed, which would be the sum of the numbers from 1 to 10 (i.e. 55).
阅读全文
相关推荐
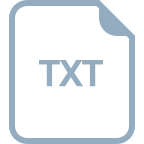
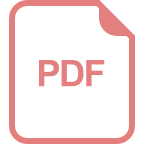
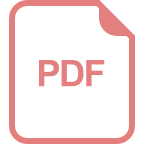













