定义学生结构体struct Student类型如下: char id[10]; //学号 char Name[20];//姓名 double Score[3];//3门课程成绩 定义并初始化struct Student数组stus[10],显示stus数组数据,输入学生学号,查找该生的数据;删除符合某个条件的所有学生数据;建立struct Student类型的变量并赋值,将该变量插入到stus数组的末尾。用c语言编写
时间: 2024-01-23 11:02:24 浏览: 28
以下是你需要的代码:
```c
#include <stdio.h>
#include <string.h>
struct Student {
char id[10]; //学号
char Name[20]; //姓名
double Score[3]; //3门课程成绩
};
void display(struct Student stus[], int size) {
printf("学号\t姓名\t成绩1\t成绩2\t成绩3\n");
for (int i = 0; i < size; i++) {
printf("%s\t%s\t%.2lf\t%.2lf\t%.2lf\n", stus[i].id, stus[i].Name, stus[i].Score[0], stus[i].Score[1], stus[i].Score[2]);
}
}
int find(struct Student stus[], int size, char id[]) {
for (int i = 0; i < size; i++) {
if (strcmp(stus[i].id, id) == 0) {
return i;
}
}
return -1;
}
int remove_by_condition(struct Student stus[], int size, double condition) {
int count = 0;
for (int i = 0; i < size; i++) {
if (stus[i].Score[0] == condition || stus[i].Score[1] == condition || stus[i].Score[2] == condition) {
for (int j = i; j < size - 1; j++) {
stus[j] = stus[j + 1];
}
count++;
size--;
i--;
}
}
return count;
}
int main() {
struct Student stus[10] = {
{"1001", "张三", {90.5, 80.5, 70.5}},
{"1002", "李四", {70.5, 60.5, 90.5}},
{"1003", "王五", {80.5, 70.5, 60.5}},
{"1004", "赵六", {60.5, 90.5, 80.5}},
{"1005", "钱七", {90.5, 70.5, 80.5}}
};
int size = 5;
display(stus, size);
char id[10];
printf("请输入学号:");
scanf("%s", id);
int index = find(stus, size, id);
if (index == -1) {
printf("未找到该学号的学生\n");
} else {
printf("学号\t姓名\t成绩1\t成绩2\t成绩3\n");
printf("%s\t%s\t%.2lf\t%.2lf\t%.2lf\n", stus[index].id, stus[index].Name, stus[index].Score[0], stus[index].Score[1], stus[index].Score[2]);
}
double condition;
printf("请输入要删除的成绩:");
scanf("%lf", &condition);
int count = remove_by_condition(stus, size, condition);
size -= count;
display(stus, size);
struct Student new_stu = {"1006", "孙八", {85.5, 95.5, 75.5}};
stus[size] = new_stu;
size++;
display(stus, size);
return 0;
}
```
在这个程序中,我们首先定义了结构体 `Student`,包括学号、姓名和三门课程的成绩。然后我们定义了一个数组 `stus`,用于存储学生的数据。
在 `main` 函数中,我们首先调用 `display` 函数来显示所有学生的数据,然后通过 `find` 函数来查找指定学号的学生数据,并输出。
接下来,我们调用 `remove_by_condition` 函数来删除符合指定条件的所有学生数据,并输出剩余的学生数据。
最后,我们定义了一个新的学生变量 `new_stu`,并将其插入到 `stus` 数组的末尾,然后再次调用 `display` 函数来显示所有学生的数据。
相关推荐
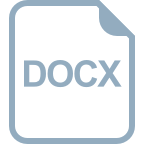
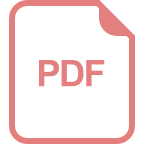
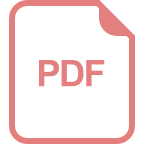




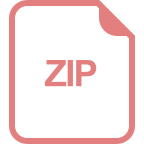
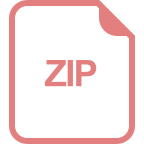
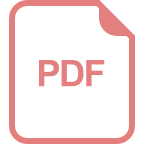
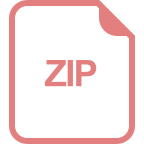
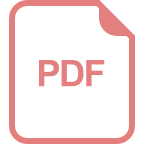
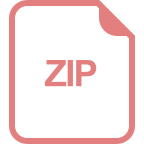
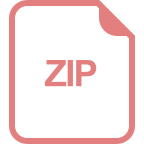
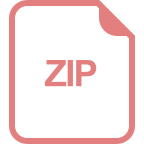
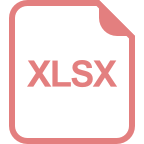
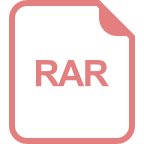
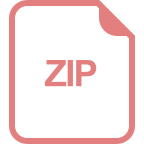