uni-app实现点击div就添加背景图片
时间: 2023-08-03 13:08:56 浏览: 98
要实现点击 div 就添加背景图片,可以通过以下步骤实现:
1. 在 div 中添加一个点击事件,可以使用 v-on:click 或 @click 绑定事件。
2. 在事件处理函数中,获取需要设置背景图片的 div 元素,可以使用 $refs 属性获取。
3. 使用样式绑定的方式,将背景图片设置为 div 元素的背景图片。
下面是一个示例代码:
```html
<template>
<div ref="myDiv" @click="addBackgroundImage"></div>
</template>
<script>
export default {
methods: {
addBackgroundImage() {
const myDiv = this.$refs.myDiv;
myDiv.style.backgroundImage = "url('/images/background.jpg')";
},
},
};
</script>
<style>
.my-div {
width: 200px;
height: 200px;
}
</style>
```
在上面的代码中,myDiv 变量获取了 div 元素,然后设置了其背景图片为 /images/background.jpg。你可以根据实际需求,修改代码中的图片路径和样式。
相关问题
uni-app实现背景遮罩不能触碰
可以通过在背景遮罩上添加一个阻止触摸事件的 div 元素来实现。具体步骤如下:
1. 在页面中添加一个背景遮罩元素,并设置其 z-index 值比其他元素小,以覆盖整个页面。
2. 在背景遮罩元素上添加一个绝对定位的 div 元素,并设置其宽高为 100%,并设置 z-index 值比背景遮罩元素大。
3. 在 div 元素上添加一个阻止触摸事件的 touchstart 或 touchmove 事件,代码如下:
```javascript
document.querySelector('.mask .disable-touch').addEventListener('touchstart', function(e) {
e.preventDefault();
}, {passive: false});
document.querySelector('.mask .disable-touch').addEventListener('touchmove', function(e) {
e.preventDefault();
}, {passive: false});
```
这样,当用户触摸背景遮罩时,所有事件都会被阻止,从而实现了背景遮罩不能触碰的效果。
uni-app 之uni-td 点击事件
对于uni-app中的uni-td组件,它是用于展示表格数据的组件,并不支持直接绑定点击事件。但是你可以通过给uni-td组件添加一个父级元素,并为父级元素绑定点击事件来实现类似的功能。
首先,在你的uni-app页面中,添加一个父级元素,例如一个div标签,然后给这个div标签绑定点击事件,如下所示:
```html
<template>
<div @tap="handleClick">
<uni-td>{{ data }}</uni-td>
</div>
</template>
```
在上面的代码中,我们给div标签绑定了一个点击事件`@tap="handleClick"`,当用户点击这个div标签时,就会触发`handleClick`方法。
接下来,在同一个页面的`<script>`标签中,定义`handleClick`方法来处理点击事件:
```javascript
<script>
export default {
methods: {
handleClick() {
// 处理点击事件的逻辑
console.log('点击了uni-td');
}
}
}
</script>
```
在上面的代码中,我们定义了一个`handleClick`方法,在方法内部可以处理你需要的点击事件的逻辑。例如,在这个方法中,我们使用`console.log`打印了一条消息"点击了uni-td"。
这样,当用户点击uni-td组件所在的父级div标签时,就会触发`handleClick`方法,并执行其中的逻辑。
希望能帮到你!如有任何疑问,请随时追问。
相关推荐
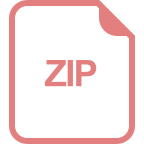
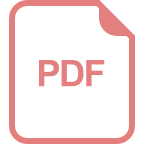












