用python进行lstm滚动预测
时间: 2024-01-26 13:01:52 浏览: 41
以下是一个简单的 Python LSTM 滚动预测示例:
1. 导入必要的库
```
import numpy as np
from keras.models import Sequential
from keras.layers import Dense, LSTM
```
2. 生成数据
假设我们有一组包含 100 个值的时间序列数据,现在我们需要将其转换为 LSTM 模型可以处理的格式。我们可以使用滚动窗口方法,将每个时刻的前 3 个数据作为输入,第 4 个数据作为输出。
```
# 生成数据
data = [i*np.sin(i/10) for i in range(100)]
sequence_length = 4
result = []
for i in range(len(data)-sequence_length):
result.append(data[i:i+sequence_length])
# 将数据转换为 numpy 数组
result = np.array(result)
print(result.shape) # 输出 (96, 4)
```
3. 划分数据集
将数据集划分为训练集和测试集。我们可以将前 70% 的数据用于训练,后 30% 的数据用于测试。
```
# 划分数据集
row = int(round(result.shape[0] * 0.7))
train = result[:row, :]
np.random.shuffle(train)
x_train = train[:, :-1]
y_train = train[:, -1]
x_test = result[row:, :-1]
y_test = result[row:, -1]
```
4. 构建模型
使用 Keras 构建 LSTM 模型。
```
# 构建模型
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(3, 1)))
model.add(LSTM(units=50))
model.add(Dense(units=1))
model.compile(optimizer='adam', loss='mean_squared_error')
```
5. 训练模型
```
# 训练模型
x_train = np.reshape(x_train, (x_train.shape[0], x_train.shape[1], 1))
model.fit(x_train, y_train, epochs=100, batch_size=32)
```
6. 测试模型
使用测试集来评估模型的性能。
```
# 测试模型
x_test = np.reshape(x_test, (x_test.shape[0], x_test.shape[1], 1))
predictions = model.predict(x_test)
```
7. 可视化结果
将原始数据和模型预测结果可视化。
```
import matplotlib.pyplot as plt
plt.plot(y_test, label='True')
plt.plot(predictions, label='Predicted')
plt.legend()
plt.show()
```
这将显示原始数据和 LSTM 模型的预测结果。
相关推荐
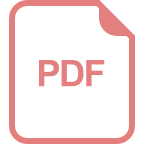
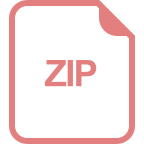














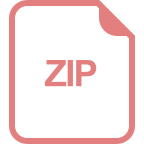