用java针对学生选课系统写一个测试类,输入1展示课程信息,输入2进行选课,输入3查看个人选课,且当选中一个课程时,该课程标注为已选,选课人数加一
时间: 2024-03-03 18:50:44 浏览: 10
好的,我可以为您编写这个测试类。首先,我们需要先定义一个 `Course` 类和一个 `Student` 类,代码如下:
```java
public class Course {
private String name;
private int capacity;
private int enrolled;
public Course(String name, int capacity) {
this.name = name;
this.capacity = capacity;
this.enrolled = 0;
}
public String getName() {
return name;
}
public int getCapacity() {
return capacity;
}
public int getEnrolled() {
return enrolled;
}
public boolean isFull() {
return enrolled >= capacity;
}
public void enroll() {
enrolled++;
}
}
public class Student {
private String name;
private List<Course> courses;
public Student(String name) {
this.name = name;
this.courses = new ArrayList<>();
}
public String getName() {
return name;
}
public List<Course> getCourses() {
return courses;
}
public void enroll(Course course) {
if (!course.isFull()) {
course.enroll();
courses.add(course);
} else {
System.out.println("Sorry, the course is already full.");
}
}
}
```
接下来,我们可以编写一个名为 `CourseRegistrationTest` 的测试类,来测试这个选课系统。代码如下:
```java
import java.util.Scanner;
public class CourseRegistrationTest {
public static void main(String[] args) {
Course[] courses = {
new Course("Java Programming", 50),
new Course("Data Structures and Algorithms", 40),
new Course("Web Development", 30)
};
Student student = new Student("Alice");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("1. Show course information");
System.out.println("2. Enroll in a course");
System.out.println("3. View personal course schedule");
System.out.print("Please enter your choice (1-3): ");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
for (Course course : courses) {
System.out.printf("%s (%d/%d)\n", course.getName(), course.getEnrolled(), course.getCapacity());
}
break;
case 2:
System.out.print("Please enter the name of the course: ");
String courseName = scanner.nextLine();
boolean foundCourse = false;
for (Course course : courses) {
if (course.getName().equals(courseName)) {
foundCourse = true;
student.enroll(course);
break;
}
}
if (!foundCourse) {
System.out.println("Sorry, the course is not found.");
}
break;
case 3:
System.out.println("Personal course schedule:");
for (Course course : student.getCourses()) {
System.out.println(course.getName());
}
break;
default:
System.out.println("Invalid choice. Please try again.");
break;
}
System.out.println();
}
}
}
```
这个测试类首先创建了三个课程,并创建一个名为 `Alice` 的学生。然后,它进入一个循环,让用户输入选项。如果用户输入 `1`,它会展示所有课程的信息,包括课程名称和已选人数。如果用户输入 `2`,它会提示用户输入课程名称,并尝试让学生选修这个课程。如果用户输入 `3`,它会展示学生已选的课程。如果用户输入无效的选项,它会提示用户重新输入。注意,当课程已经满员时,学生无法再选修该课程。
希望这个测试类能够满足您的需求。如果您有任何问题,请随时问我。
相关推荐
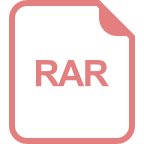
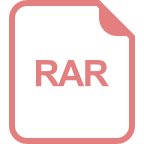















