c++写一段判断分别由两个xy坐标表示的两条线是否相交,如相交输出交点坐标的函数
时间: 2023-08-13 08:07:39 浏览: 133
可以使用向量叉积的方法来判断两条线段是否相交,如果相交则可以求出交点的坐标。具体实现如下:
```c++
#include <iostream>
#include <cmath>
using namespace std;
struct Point {
double x, y;
};
// 向量叉积,返回向量AB和向量AC的叉积
double crossProduct(Point A, Point B, Point C) {
return (B.x - A.x) * (C.y - A.y) - (B.y - A.y) * (C.x - A.x);
}
// 判断线段AB和CD是否相交
bool isIntersect(Point A, Point B, Point C, Point D) {
double c1 = crossProduct(A, B, C);
double c2 = crossProduct(A, B, D);
double c3 = crossProduct(C, D, A);
double c4 = crossProduct(C, D, B);
if (c1 * c2 < 0 && c3 * c4 < 0) {
return true;
}
return false;
}
// 求线段AB和CD的交点坐标
Point getIntersection(Point A, Point B, Point C, Point D) {
double k1 = (B.y - A.y) / (B.x - A.x);
double b1 = A.y - k1 * A.x;
double k2 = (D.y - C.y) / (D.x - C.x);
double b2 = C.y - k2 * C.x;
double x = (b2 - b1) / (k1 - k2);
double y = k1 * x + b1;
return {x, y};
}
int main() {
Point A = {1, 1};
Point B = {4, 4};
Point C = {1, 4};
Point D = {4, 1};
if (isIntersect(A, B, C, D)) {
Point P = getIntersection(A, B, C, D);
cout << "Intersection point is (" << P.x << ", " << P.y << ")" << endl;
} else {
cout << "The two lines do not intersect." << endl;
}
return 0;
}
```
上述代码中,`isIntersect`函数用来判断两条线段是否相交,它利用向量叉积的性质来判断。如果两个向量的叉积的乘积小于零,则表示这两个向量的方向相反,即线段相交。
`getIntersection`函数用来求出两条线段的交点坐标,它首先求出两条直线的斜率和截距,然后求出交点的横坐标和纵坐标。
在上述代码中,我们假设线段AB和CD不平行和共线。如果需要处理这些特殊情况,可以根据具体需求进行修改。
阅读全文
相关推荐
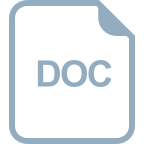
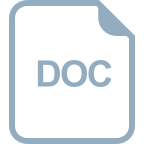
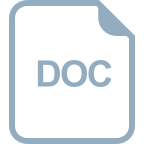



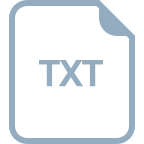
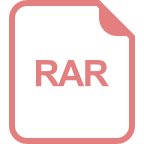
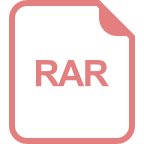
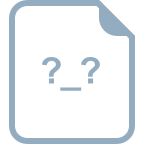
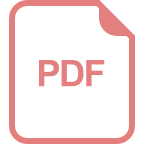
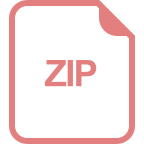
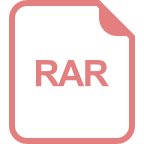
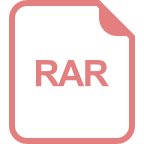
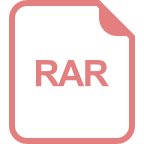
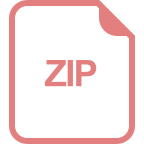
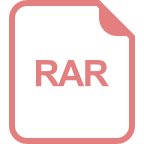
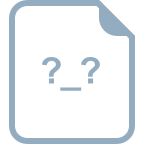