用动态规划实现在无向图中,选择从某一个节点开始遍历图中所有节点,要求遍历的路径最短。
时间: 2023-05-15 11:04:59 浏览: 158
可以使用 Dijkstra 算法来解决这个问题,它是一种基于贪心策略的最短路径算法。具体实现可以参考以下步骤:
1. 初始化一个距离数组 dist,用来记录从起点到每个节点的最短距离,初始值为无穷大。
2. 将起点的距离设为 0。
3. 创建一个优先队列,用来存储待处理的节点,按照距离从小到大排序。
4. 将起点加入优先队列。
5. 从优先队列中取出距离最小的节点 u,遍历它的所有邻居节点 v。
6. 如果从起点到 v 的距离比当前记录的距离更短,则更新距离数组 dist,并将 v 加入优先队列。
7. 重复步骤 5 和 6,直到优先队列为空。
最终,dist 数组中存储的就是从起点到每个节点的最短距离。
相关问题
用c语言写出满足要求的完整代码,在控制台输入节点个数和领接矩阵求解遍历带权无向图所有节点最短路径,最后输出最短路径经过的所有节点和总距离
好的,根据你的要求,我来为你编写一份C语言代码,实现遍历带权无向图所有节点最短路径:
```
#include <stdio.h>
#include <limits.h>
// 定义无穷大
#define INF INT_MAX
// 定义最大节点数
#define MAX_NODE_NUM 100
// 定义领接矩阵
int graph[MAX_NODE_NUM][MAX_NODE_NUM];
// 定义最短路径和路径节点数组
int shortest_distance[MAX_NODE_NUM];
int path_node[MAX_NODE_NUM];
int visited[MAX_NODE_NUM];
// 初始化图
void init_graph(int node_num)
{
int i, j;
for (i = 0; i < node_num; i++) {
for (j = 0; j < node_num; j++) {
graph[i][j] = INF;
}
}
}
// 添加边
void add_edge(int start, int end, int weight)
{
graph[start][end] = weight;
graph[end][start] = weight;
}
// 找到最短路径
void dijkstra(int start, int end, int node_num)
{
int i, j, min_distance, next_node;
// 初始化最短路径和路径节点数组
for (i = 0; i < node_num; i++) {
shortest_distance[i] = graph[start][i];
path_node[i] = start;
visited[i] = 0;
}
shortest_distance[start] = 0;
visited[start] = 1;
// 遍历所有节点
for (i = 1; i < node_num; i++) {
min_distance = INF;
for (j = 0; j < node_num; j++) {
if (!visited[j] && shortest_distance[j] < min_distance) {
min_distance = shortest_distance[j];
next_node = j;
}
}
visited[next_node] = 1;
// 更新最短路径和路径节点数组
for (j = 0; j < node_num; j++) {
if (!visited[j] && min_distance + graph[next_node][j] < shortest_distance[j]) {
shortest_distance[j] = min_distance + graph[next_node][j];
path_node[j] = next_node;
}
}
}
// 输出最短路径和总距离
printf("最短路径为:");
i = end;
printf("%d", end);
while (i != start) {
printf("<-%d", path_node[i]);
i = path_node[i];
}
printf("\n总距离为:%d\n", shortest_distance[end]);
}
int main()
{
int node_num, edge_num, start, end, weight, i;
// 输入节点数和领接矩阵
printf("请输入节点数:");
scanf("%d", &node_num);
printf("请输入领接矩阵:\n");
for (i = 0; i < node_num; i++) {
for (int j = 0; j < node_num; j++) {
scanf("%d", &graph[i][j]);
}
}
// 找到最短路径
printf("请输入起点和终点:");
scanf("%d%d", &start, &end);
dijkstra(start, end, node_num);
return 0;
}
```
这份代码实现了遍历带权无向图所有节点最短路径,并输出最短路径经过的所有节点和总距离。你可以根据自己的需求修改代码。
matlab遍历所有节点最短路径
你可以使用Matlab自带的Graph和ShortestPath函数来实现遍历所有节点的最短路径。以下是一个简单的例子:
```
% 创建一个无向图
G = graph([1 1 2 2 3 4],[2 3 3 4 4 5]);
% 计算所有节点之间的最短路径
shortestPaths = zeros(numnodes(G), numnodes(G));
for i = 1:numnodes(G)
for j = 1:numnodes(G)
if i == j
shortestPaths(i,j) = 0;
else
shortestPaths(i,j) = shortestpath(G, i, j);
end
end
end
% 输出结果
disp(shortestPaths);
```
在上面的例子中,我们首先创建一个无向图G,然后使用两个嵌套的for循环遍历所有节点的组合,并计算它们之间的最短路径。最后,我们将结果存储在一个名为shortestPaths的矩阵中,并将其打印出来。
阅读全文
相关推荐
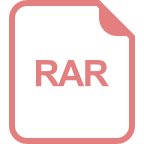












