给出一个python实现的MCTS算法代码
时间: 2023-02-17 09:08:28 浏览: 74
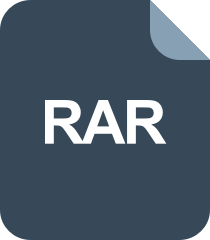
算法领域python实现代码
下面是一个简单的MCTS算法实现,使用Python语言实现:
```python
import math
import random
class Node:
def __init__(self, state, parent=None):
self.state = state
self.parent = parent
self.children = []
self.wins = 0
self.visits = 0
def add_child(self, child_state):
child = Node(child_state, self)
self.children.append(child)
return child
def update(self, result):
self.visits += 1
self.wins += result
def fully_expanded(self):
return len(self.children) == len(self.state.get_moves())
def __repr__(self):
return "[M:" + str(self.wins/self.visits) + " W/V:" + str(self.wins) + "/" + str(self.visits) + "]"
def tree_to_string(self, indent):
s = self.indent_string(indent) + str(self)
for c in self.children:
s += c.tree_to_string(indent+1)
return s
def indent_string(self, indent):
s = "\n"
for i in range (1, indent+1):
s += "| "
return s
def child_N(self):
s = self.indent_string(1) + "children:" + "\n"
for c in self.children:
s += str(c.wins/c.visits) + ","
return s
class MCTS:
def __init__(self, state, itermax):
self.itermax = itermax
self.root = Node(state)
def search(self):
for i in range(self.itermax):
node = self.select_node(self.root)
result = self.simulate(node)
self.backpropagate(node, result)
return self.get_best_child(self.root)
def select_node(self, node):
while not node.state.is_terminal():
if not node.fully_expanded():
return self.expand(node)
else:
node = self.get_best_child(node)
return node
def expand(self, node):
tries = node.state.get_moves()
for move in tries:
if move not in [n.state for n in node.children]:
new_node = node.add_child(node.state.do_move(move))
return new_node
def simulate(self, node):
state = node.state
while not state.is_terminal():
state = state.do_move(random.choice(state.get_moves()))
return state.get_result(node.state.player_just_moved)
def backpropagate(self, node
阅读全文
相关推荐







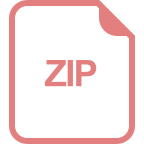
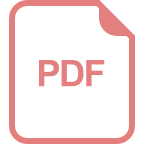
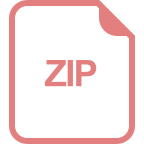
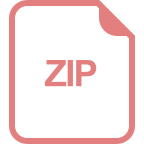
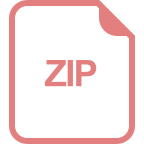
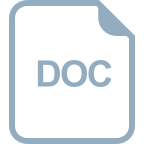
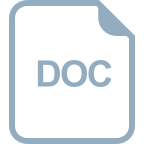
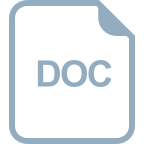
