Unity代码删除物体上的组件
时间: 2024-03-23 08:39:57 浏览: 26
在Unity中,你也可以通过代码来删除物体上的组件。以下是一个示例代码:
```
// 获取物体上的指定组件
Component component = gameObject.GetComponent<ComponentType>();
// 如果该组件存在,则删除
if (component != null)
{
Destroy(component);
}
```
这段代码首先使用`GetComponent<ComponentType>()`方法获取物体上的指定组件,如`Transform`、`Rigidbody`等。如果该组件存在,则使用`Destroy(component)`方法将其销毁。注意,这种方法只能在运行时删除组件,并且只能删除已存在的组件。如果要动态添加和删除组件,可以使用`AddComponent<ComponentType>()`方法来添加组件,使用`DestroyImmediate(component)`方法来立即销毁组件。
相关问题
Unity 静态类中用代码删除物体上的组件
在Unity中,你可以在静态类中使用代码删除物体上的组件。以下是一个示例代码:
```
public static class ComponentUtils
{
public static void RemoveComponent<T>(GameObject gameObject) where T : Component
{
T component = gameObject.GetComponent<T>();
if (component != null)
{
Object.Destroy(component);
}
}
}
```
这段代码定义了一个名为`ComponentUtils`的静态类,其中包含一个名为`RemoveComponent`的静态方法。该方法接受一个类型参数`T`,表示要删除的组件类型,以及一个`GameObject`对象,表示要从中删除组件的物体。
在方法中,首先使用`GetComponent<T>()`方法获取指定类型的组件。如果该组件存在,则使用`Destroy(component)`方法将其销毁。注意,这里使用的是`Object.Destroy(component)`,而不是直接调用`Destroy(component)`。这是因为在静态类中无法直接访问`UnityEngine.Object`类,需要在方法中显式地引用。
使用这个静态方法时,你可以像这样调用它:
```
ComponentUtils.RemoveComponent<Rigidbody>(gameObject);
```
这将从`gameObject`对象中删除`Rigidbody`组件(如果存在)。
unity怎么用脚本将物体上的脚本删除
要在 Unity 中使用脚本删除物体上的脚本,你可以使用 `Destroy()` 方法。以下是一个示例代码:
```csharp
// 获取物体上的脚本组件
YourScript yourScript = targetObject.GetComponent<YourScript>();
// 如果脚本组件存在,则将其销毁
if (yourScript != null)
{
Destroy(yourScript);
}
```
在这个示例中,`targetObject` 是你想要删除脚本的目标物体,`YourScript` 是你要删除的脚本的类型。首先,通过使用 `GetComponent<YourScript>()` 方法获取目标物体上的脚本组件的引用。然后,使用 `Destroy()` 方法将脚本组件销毁。
请注意,这只会删除脚本组件,而不会删除物体本身。如果你想要同时删除物体和脚本,可以使用 `Destroy(targetObject)` 方法。
希望能解答你的问题!如有其他疑问,请随时提问。
相关推荐
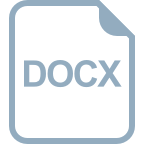
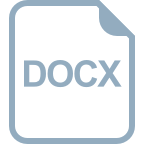












