Python中对文件中某一类进行分类
时间: 2023-12-06 14:45:07 浏览: 81
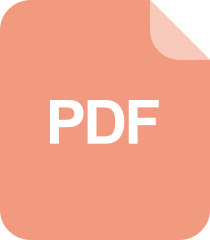
python实现根据文件格式分类
可以通过读取文件内容并根据特定规则进行分析和分类,例如:
1. 根据文件后缀名进行分类:
```python
import os
# 指定文件夹路径和要分类的后缀名
folder_path = './files'
file_ext = '.txt'
# 遍历文件夹,将指定后缀名的文件移动到对应分类的文件夹中
for file_name in os.listdir(folder_path):
if file_name.endswith(file_ext):
file_path = os.path.join(folder_path, file_name)
new_folder = os.path.join(folder_path, file_ext[1:])
if not os.path.exists(new_folder):
os.makedirs(new_folder)
os.rename(file_path, os.path.join(new_folder, file_name))
```
2. 根据文件内容进行分类:
```python
import os
# 指定文件夹路径和分类规则
folder_path = './files'
rules = {
'python': ['python', 'django', 'flask'],
'javascript': ['javascript', 'react', 'vue'],
'html': ['html', 'css', 'bootstrap']
}
# 遍历文件夹,根据分类规则将文件移动到对应分类的文件夹中
for file_name in os.listdir(folder_path):
file_path = os.path.join(folder_path, file_name)
with open(file_path, 'r') as f:
content = f.read()
for category, keywords in rules.items():
for keyword in keywords:
if keyword in content.lower():
new_folder = os.path.join(folder_path, category)
if not os.path.exists(new_folder):
os.makedirs(new_folder)
os.rename(file_path, os.path.join(new_folder, file_name))
```
阅读全文
相关推荐
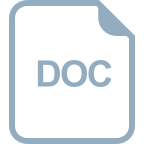


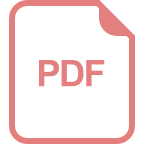
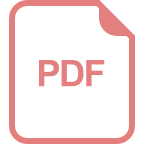
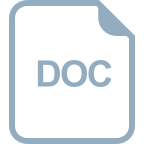








