2、 编写C语言程序实现以下功能:编写一个通讯录读写程序,要求使用fcntl()函数给通讯录数据文件加文件记录锁,实现数据库的“行级锁”。通讯录数据文件格式:编号<Tab>姓名<Tab>手机<Tab>固定电话<Tab>地址<Tab> contack.data
时间: 2023-06-20 14:08:00 浏览: 327
以下是一个基本实现通讯录读写程序的C语言代码,包含了使用fcntl()函数加文件记录锁实现“行级锁”的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
#define MAX_CONTACTS 1000
#define RECORD_SIZE 256
struct Contact {
int id;
char name[32];
char phone[16];
char tel[16];
char address[64];
};
int main() {
struct Contact contacts[MAX_CONTACTS];
int num_contacts = 0;
int fd = open("contack.data", O_RDWR | O_CREAT, 0644);
if (fd == -1) {
perror("open");
exit(1);
}
// 加文件锁,实现“行级锁”
struct flock fl;
fl.l_type = F_WRLCK;
fl.l_whence = SEEK_SET;
fl.l_start = 0;
fl.l_len = 0;
fl.l_pid = getpid();
if (fcntl(fd, F_SETLKW, &fl) == -1) {
perror("fcntl");
exit(1);
}
// 读取通讯录数据
char record[RECORD_SIZE];
while (read(fd, record, RECORD_SIZE) == RECORD_SIZE) {
char *token = strtok(record, "\t");
contacts[num_contacts].id = atoi(token);
token = strtok(NULL, "\t");
strcpy(contacts[num_contacts].name, token);
token = strtok(NULL, "\t");
strcpy(contacts[num_contacts].phone, token);
token = strtok(NULL, "\t");
strcpy(contacts[num_contacts].tel, token);
token = strtok(NULL, "\t");
strcpy(contacts[num_contacts].address, token);
num_contacts++;
}
// 添加或修改通讯录数据
struct Contact new_contact;
printf("请输入编号:");
scanf("%d", &new_contact.id);
printf("请输入姓名:");
scanf("%s", new_contact.name);
printf("请输入手机:");
scanf("%s", new_contact.phone);
printf("请输入固定电话:");
scanf("%s", new_contact.tel);
printf("请输入地址:");
scanf("%s", new_contact.address);
int found = 0;
for (int i = 0; i < num_contacts; i++) {
if (contacts[i].id == new_contact.id) {
memcpy(&contacts[i], &new_contact, sizeof(struct Contact));
found = 1;
break;
}
}
if (!found) {
memcpy(&contacts[num_contacts], &new_contact, sizeof(struct Contact));
num_contacts++;
}
// 写入通讯录数据
lseek(fd, 0, SEEK_SET);
for (int i = 0; i < num_contacts; i++) {
sprintf(record, "%d\t%s\t%s\t%s\t%s\n", contacts[i].id, contacts[i].name, contacts[i].phone, contacts[i].tel, contacts[i].address);
write(fd, record, strlen(record));
}
// 释放文件锁
fl.l_type = F_UNLCK;
if (fcntl(fd, F_SETLK, &fl) == -1) {
perror("fcntl");
exit(1);
}
close(fd);
return 0;
}
```
该程序首先使用open()函数打开或创建通讯录数据文件,并使用fcntl()函数加文件记录锁。然后读取通讯录数据,将其存储在一个结构体数组中,用户可以输入新的通讯录数据,程序会在结构体数组中查找是否已存在该编号的记录,如果存在则修改,否则添加新的记录。最后将结构体数组中的数据写入文件,并使用fcntl()函数释放文件记录锁。
注意,该程序没有进行任何输入合法性检查,实际应用中需要对用户输入进行检查和验证。同时,该程序只是演示了如何使用fcntl()函数实现“行级锁”,实际应用中需要考虑并发访问和多线程安全等问题。
相关推荐
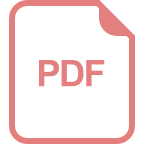
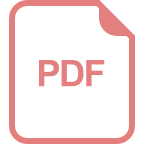
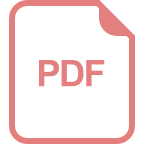














