请给我在unity2d中,实现点击画面中的文件,捡起多个文件,并可以用按钮切换文件,并且可以将各个文件上的照片拖拽到墙上的正确位置的代码。当所有文件上的照片拖拽结束后,返回桌面的按钮激活的代码
时间: 2024-05-04 21:15:25 浏览: 152
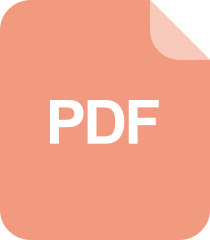
javascript实现了照片拖拽点击置顶的照片墙代码
以下是可能的实现方式:
1. 定义一个文件类(File),其中包含照片和文件名等属性。
2. 在场景中放置多个文件的GameObject,并将它们的tag设置为“File”。
3. 在脚本中监听鼠标点击事件,如果点击到了tag为“File”的GameObject,则捡起该文件,并将其加入一个文件列表中。
4. 在画面上放置多个按钮,用于切换当前选择的文件。
5. 在画面上放置一个墙的GameObject,并为其添加一个BoxCollider2D组件,表示墙的碰撞范围。
6. 在脚本中监听鼠标拖拽事件,如果当前选择的文件不为空且鼠标拖拽的位置在墙的碰撞范围内,则将该文件的照片拖拽到墙上的正确位置。
7. 当所有文件的照片拖拽结束后,返回桌面的按钮激活。
以下是可能的代码实现:
```csharp
public class File
{
public string name;
public Sprite photo;
}
public class Desktop : MonoBehaviour
{
public GameObject wall;
public Button returnButton;
public List<File> files = new List<File>();
public int currentFileIndex = -1;
private File currentFile;
private void Start()
{
returnButton.onClick.AddListener(ReturnToDesktop);
}
private void Update()
{
if (Input.GetMouseButtonDown(0))
{
RaycastHit2D hit = Physics2D.Raycast(Camera.main.ScreenToWorldPoint(Input.mousePosition), Vector2.zero);
if (hit.collider != null && hit.collider.tag == "File")
{
File file = new File();
file.name = hit.collider.gameObject.name;
file.photo = hit.collider.gameObject.GetComponent<SpriteRenderer>().sprite;
files.Add(file);
hit.collider.gameObject.SetActive(false);
if (currentFileIndex < 0)
{
SetCurrentFile(0);
}
}
}
}
private void OnMouseDrag()
{
if (currentFile != null)
{
Vector3 position = Camera.main.ScreenToWorldPoint(Input.mousePosition);
position.z = 0;
if (wall.GetComponent<BoxCollider2D>().OverlapPoint(position))
{
currentFile.photo.transform.position = position;
}
}
}
private void SetCurrentFile(int index)
{
if (currentFileIndex >= 0)
{
currentFile.photo.gameObject.SetActive(false);
}
currentFileIndex = index;
currentFile = files[currentFileIndex];
currentFile.photo.gameObject.SetActive(true);
}
private void ReturnToDesktop()
{
foreach (File file in files)
{
file.photo.gameObject.SetActive(true);
}
files.Clear();
currentFileIndex = -1;
currentFile = null;
}
}
```
阅读全文
相关推荐
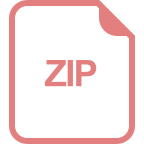



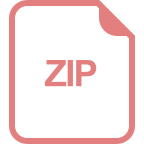
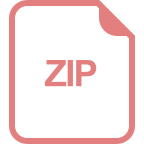
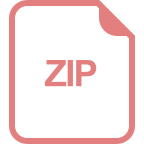
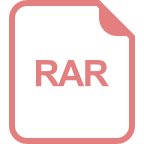
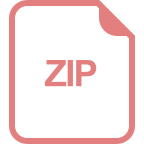
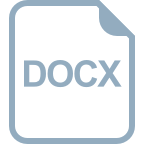
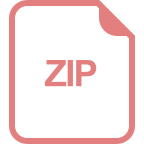
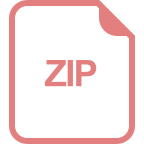
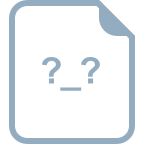
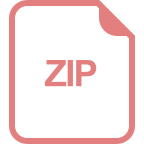
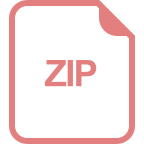
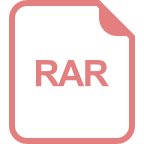
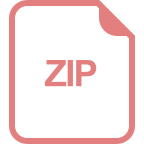