with torch.no_grad():
时间: 2023-04-28 21:04:17 浏览: 113
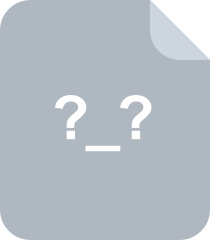
0695-极智开发-解读pytorch中with torch.no-grad()的作用
`torch.no_grad()` 是 PyTorch 中一个上下文管理器,在进入这个上下文环境后,PyTorch 不会记录对变量的任何操作,也不会计算梯度,这样可以节省内存和计算时间。常用于测试代码或评估模型时。
举个例子
```
with torch.no_grad():
x = torch.randn(3, requires_grad=True)
y = x * 2
print(y.requires_grad) # False
```
这里我们用with torch.no_grad()禁止跟踪对tensor的操作,对于y来说也不需要求导,y.requires_grad 就是false
通常我们在评估模型时使用这个上下文管理器。
```
with torch.no_grad():
model.eval()
output = model(input)
loss = criterion(output, target)
```
在评估模型时不需要求导,我们可以使用 `torch.no_grad()` 上下文管理器,跳过计算梯度,从而提高计算速度和节省内存。
阅读全文
相关推荐
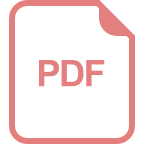
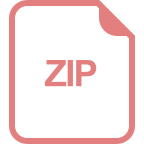


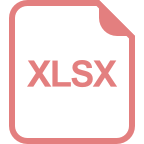
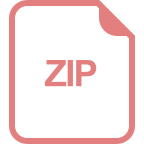
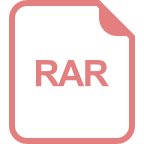
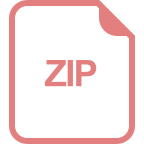
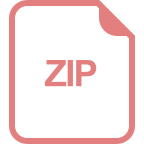
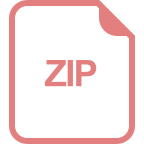
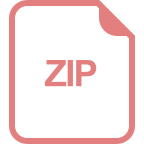
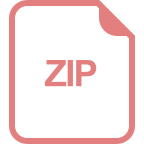
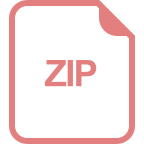
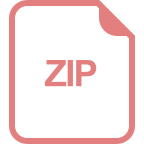
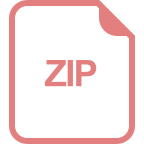
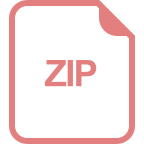