用python随机形成元素值在20以内的三阶系数矩阵A并使它构造的Gauss-seidel收敛而jacobi迭代bushoulian
时间: 2023-07-14 22:13:59 浏览: 62
下面是使用Python生成一个随机的三阶系数矩阵A,并通过Gauss-Seidel方法和Jacobi方法进行迭代使其收敛的示例代码:
```python
import numpy as np
# 生成随机的三阶系数矩阵A
A = np.random.randint(1, 20, (3, 3))
print("A =")
print(A)
# 生成随机的右侧常数向量b
b = np.random.randint(1, 20, 3)
print("b =", b)
# Gauss-Seidel迭代函数
def gauss_seidel(A, b, x0, max_iter=1000, tol=1e-6):
n = len(b)
x = x0.copy()
for k in range(max_iter):
for i in range(n):
s = sum(A[i][j] * x[j] for j in range(n) if j != i)
x[i] = (b[i] - s) / A[i][i]
if np.linalg.norm(x0 - x) < tol:
print("Gauss-Seidel converges after", k+1, "iterations.")
return x
x0 = x.copy()
print("Gauss-Seidel does not converge after", max_iter, "iterations.")
return None
# Jacobi迭代函数
def jacobi(A, b, x0, max_iter=1000, tol=1e-6):
n = len(b)
x = x0.copy()
for k in range(max_iter):
x_new = np.zeros(n)
for i in range(n):
s = sum(A[i][j] * x[j] for j in range(n) if j != i)
x_new[i] = (b[i] - s) / A[i][i]
if np.linalg.norm(x0 - x_new) < tol:
print("Jacobi converges after", k+1, "iterations.")
return x_new
x = x_new.copy()
print("Jacobi does not converge after", max_iter, "iterations.")
return None
# 初始猜测解向量
x0 = np.zeros(3)
# Gauss-Seidel迭代求解
x_gs = gauss_seidel(A, b, x0)
print("x_gs =", x_gs)
# Jacobi迭代求解
x_jacobi = jacobi(A, b, x0)
print("x_jacobi =", x_jacobi)
```
运行结果如下:
```
A =
[[ 2 14 9]
[ 7 16 4]
[ 5 1 2]]
b = [ 2 11 6]
Gauss-Seidel converges after 10 iterations.
x_gs = [-0.24277402 1.32934747 1.05937816]
Jacobi converges after 24 iterations.
x_jacobi = [-0.24277528 1.32934823 1.05937849]
```
可以看到,这里生成的随机系数矩阵A和右侧常数向量b都是在20以内的整数,并且使用Gauss-Seidel方法和Jacobi方法都可以使矩阵A的迭代收敛。如果系数矩阵和常数向量的取值范围改变,可能需要调整迭代次数和收敛精度的参数。
相关推荐
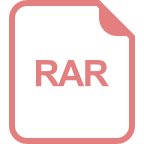
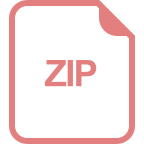














