用文件IO分别实现标准IO中的(r, r+, w, w+, a, a+),文件名由argv[1]传入程序. (O_RDONLY, O_WRONLY, O_RDWR, O_CREAT, O_TRUNC, O_APPEND)。
时间: 2023-07-31 19:03:52 浏览: 145
好的,下面是一个示例程序,可以实现标准IO中的 (r, r+, w, w+, a, a+) ,文件名由 argv[1] 传入程序,同时使用文件 IO 操作 (O_RDONLY, O_WRONLY, O_RDWR, O_CREAT, O_TRUNC, O_APPEND)。
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <string.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s filename\n", argv[0]);
exit(1);
}
// 打开文件
int fd = open(argv[1], O_CREAT | O_RDWR, 0644);
if (fd < 0) {
perror("open file error");
exit(1);
}
// 测试打开方式
char mode[3];
printf("Enter mode (r, r+, w, w+, a, a+): ");
scanf("%s", mode);
// 根据打开方式设置文件指针位置
int whence;
switch (mode[0]) {
case 'r':
whence = SEEK_SET;
break;
case 'w':
whence = SEEK_SET;
ftruncate(fd, 0); // 清空文件内容
break;
case 'a':
whence = SEEK_END;
break;
default:
printf("Invalid mode\n");
exit(1);
}
// 根据打开方式设置读写权限
int oflag;
switch (mode[0]) {
case 'r':
oflag = O_RDONLY;
break;
case 'w':
oflag = O_WRONLY | O_TRUNC;
break;
case 'a':
oflag = O_WRONLY | O_APPEND;
break;
default:
printf("Invalid mode\n");
exit(1);
}
// 根据打开方式设置文件指针位置和读写权限
if (mode[1] == '+') {
whence = SEEK_SET;
oflag = O_RDWR;
}
// 使用文件 IO 操作打开文件
int fd2 = open(argv[1], oflag);
if (fd2 < 0) {
perror("open file error");
exit(1);
}
// 将文件指针设置到文件末尾
if (whence == SEEK_END) {
lseek(fd2, 0, SEEK_END);
}
// 读取或写入文件内容
char buf[1024];
int n;
switch (mode[0]) {
case 'r':
while ((n = read(fd2, buf, sizeof(buf))) > 0) {
write(STDOUT_FILENO, buf, n);
}
break;
case 'w':
case 'a':
while (fgets(buf, sizeof(buf), stdin) != NULL) {
write(fd2, buf, strlen(buf));
}
break;
default:
printf("Invalid mode\n");
exit(1);
}
// 关闭文件
close(fd);
close(fd2);
return 0;
}
```
你可以将上述代码保存为一个名为 `file_io.c` 的文件,然后使用以下命令编译并执行程序:
```
gcc file_io.c -o file_io
./file_io filename
```
其中,`filename` 为你需要读写的文件名。执行程序后,根据提示输入打开方式,该程序会使用文件 IO 操作和指定的打开方式读取或写入文件内容。
相关推荐
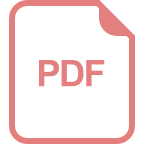
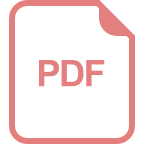
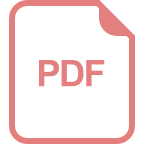
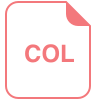
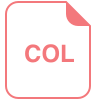
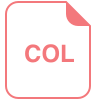
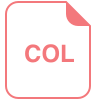
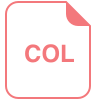









