class Employee: def __init__(self, name, id, salary): self.__name = name self.__id = id self.__salary = salary def showMessage(self): print("员工信息:") print("姓名:{};id号:{};薪金:{}元;".format(self.__name, self.__id, self.__salary))class SaleManager(Employee): def __init__(self, name, id, salary, salesRevenue, salesProfit): super().__init__(name, id, salary) self.__salesRevenue = salesRevenue self.__salesProfit = salesProfit def showMessage(self): super().showMessage() print("扩展信息:销售收入:{}元,销售利润:{}元。".format(self.__salesRevenue, self.__salesProfit))# 测试代码s = SaleManager("张三", 10001, 10000, 1000000, 100000)print("销售经理信息:")s.showMessage()
时间: 2024-01-28 09:05:13 浏览: 144
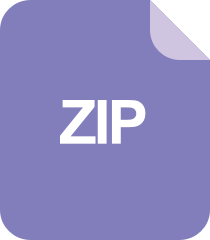
oop_workshop
这是一段 Python 代码,它展示了一个简单的面向对象编程的例子。其中定义了一个 Employee 类和一个 SaleManager 类,SaleManager 类继承了 Employee 类,并在其基础上添加了两个属性:销售收入和销售利润。同时,SaleManager 类重写了 Employee 类的 showMessage 方法,在显示员工基本信息的同时,还会显示扩展信息。
在测试代码中,我们创建了一个 SaleManager 对象 s,并调用了它的 showMessage 方法,打印出了销售经理的信息,包括基本信息和扩展信息。
阅读全文
相关推荐
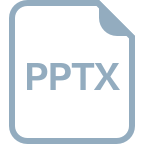
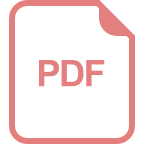















