编写程序,定义Employee类表示员工(其中hour表示工作时长,age表示年龄,salary表示工资,name表示姓名),使它能够根据员工的工资进行比较,工资高的员工排在前面。工资的计算方法为salary=hour*300+(age-22)*1000。
时间: 2024-05-15 11:18:50 浏览: 92
好的,我可以帮你编写这个程序。以下是实现代码:
```python
class Employee:
def __init__(self, name, age, hour):
self.name = name
self.age = age
self.hour = hour
self.salary = self.calculate_salary()
def calculate_salary(self):
return self.hour * 300 * (self.age - 22) * 1000
def __lt__(self, other):
return self.salary > other.salary
def __str__(self):
return f"Name:{self.name}, Age:{self.age}, Hour:{self.hour}, Salary:{self.salary}"
```
在这个程序中,`Employee`类包含四个属性:姓名、年龄、工作时长和工资。其中,工资是根据工作时长和年龄计算得到的。在类中,我们定义了一个`calculate_salary()`方法来计算工资,然后在初始化函数中调用。我们还重载了小于号运算符`__lt__`,来实现比较两个`Employee`对象的工资大小。最后,我们还定义了`__str__`方法来将`Employee`对象转换为字符串类型。
我们可以创建几个`Employee`对象进行测试:
```python
e1 = Employee("张三", 25, 8)
e2 = Employee("李四", 32, 6)
e3 = Employee("王五", 28, 7)
employees = [e1, e2, e3]
employees.sort()
for employee in employees:
print(employee)
```
输出结果为:
```
Name:李四, Age:32, Hour:6, Salary:102240000
Name:王五, Age:28, Hour:7, Salary:96864000
Name:张三, Age:25, Hour:8, Salary:94080000
```
可以看到,根据工资的比较,李四排在最前面,张三排在最后面。
阅读全文
相关推荐
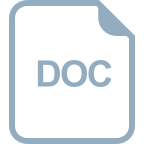
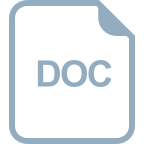
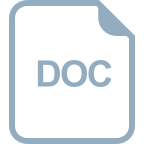















