定义一个名为Employee类表示员工,它继承Person类,要求定义一个变量salary表示员工工资。定义computeSalarydouble hours,intrate)方法计算员工工资,这里hours表示员工工作时间,rate表示每小时工资。定义默认构造方法和带name、age和salary参数的构造方法
时间: 2024-03-26 07:34:51 浏览: 60
好的,这是一个定义Employee类的Java代码,该类继承自Person类,含有一个变量salary,同时还定义了computeSalary方法:
```java
public class Employee extends Person {
private double salary;
public Employee() {
super();
}
public Employee(String name, int age, double salary) {
super(name, age);
this.salary = salary;
}
public double computeSalary(double hours, double rate) {
return hours * rate;
}
}
```
在这个类中,我们定义了一个私有属性salary来表示员工工资。我们还定义了两个构造器,一个是默认构造器,另一个是带有name、age和salary参数的构造器。其中,带有name、age和salary参数的构造器会调用父类的构造器来初始化name和age属性,而salary属性则通过参数进行初始化。最后,我们还定义了一个名为computeSalary的方法,该方法用于计算员工工资,需要传入工作时间和每小时工资作为参数,并返回计算出的工资值。
相关问题
按照下面要求定义类和创建对象 1、定于一个名为Person的类,其中含有一个String类型的成员变量name和一个int类型的成员变量age,为该类定义一个名为speak的方法,在其中输出其name和age的值。 2、为Person类定义一个带参数构造方法,通过给出姓名和年龄创建Person对象。定义默认构造方法,在该方法中调用有参数构造方法,将人的姓名设置为“张三”,年龄设置为20。 3、定义一个名为Employee类表示员工,它继承Person类,要求定义一个变量salary表示员工工资。定义computerSalary(double hours,int rate)方法计算员工工资,这里hours表示员工工作时间,rate表示每小时工资。定义默认构造方法和带name、age和salary参数的构造方法。
下面是符合要求的类定义和对象创建方法:
```java
// 定义Person类
public class Person {
private String name;
private int age;
// 默认构造方法
public Person() {
this("张三", 20);
}
// 带参数构造方法
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// speak方法
public void speak() {
System.out.println("我叫" + name + ",今年" + age + "岁。");
}
}
// 定义Employee类,继承Person类
public class Employee extends Person {
private double salary;
// 默认构造方法
public Employee() {
super();
this.salary = 0;
}
// 带name、age和salary参数的构造方法
public Employee(String name, int age, double salary) {
super(name, age);
this.salary = salary;
}
// 计算员工工资的方法
public void computeSalary(double hours, double rate) {
salary = hours * rate;
}
}
```
可以通过以下方式创建对象:
```java
// 创建Person对象
Person p = new Person();
p.speak();
// 创建Employee对象
Employee e = new Employee("李四", 30, 5000);
e.computeSalary(8, 50);
e.speak();
System.out.println("我的工资是:" + e.getSalary());
```
其中,可以通过`p.speak()`和`e.speak()`调用`Person`和`Employee`类中的`speak()`方法,输出其`name`和`age`的值;通过`e.computeSalary(8, 50)`计算员工工资;通过`e.getSalary()`获取员工工资的值。
定义一个名为employee的类,它继承person类,其中定义salary(表示工资)和department(表示部门)两个成员变量和封装这两个变量的方法。编写主程序检查新建类中的所有方法。
### 回答1:
这段代码定义了一个名为 employee 的类,它继承自 person 类。其中,定义了两个成员变量 salary(表示工资)和 department(表示部门)以及两个成员函数:一个是修改工资的方法,另一个是封装这两个变量的方法。编写主程序检查新建类中的所有方法。
### 回答2:
Employee类是一个继承Person类的类,它有两个成员变量salary和department,分别代表员工的工资和部门。在Employee类中,我们需要封装这两个成员变量,以确保它们的访问权限正确。为了实现这个目标,我们可以使用private修饰符来限制它们的访问权限,并编写公共的getter和setter方法来访问它们。
下面是一个示例Employee类:
```
public class Employee extends Person {
private double salary;
private String department;
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
}
```
在这个Employee类中,我们定义了两个成员变量salary和department,并使用private修饰符将它们封装起来。接下来,我们编写了公共的getter和setter方法来访问这两个成员变量,这样其他的类和方法就可以使用它们了。
为了检查这个新建类中的所有方法,我们可以编写一个主程序来创建一个Employee对象,并使用它的getter和setter方法来设置和获取工资和部门的值,以确保这些方法都正常工作。例如:
```
public static void main(String[] args) {
Employee employee = new Employee();
employee.setName("张三");
employee.setAge(30);
employee.setGender("男");
employee.setSalary(10000.0);
employee.setDepartment("技术部");
System.out.println("姓名:" + employee.getName());
System.out.println("年龄:" + employee.getAge());
System.out.println("性别:" + employee.getGender());
System.out.println("工资:" + employee.getSalary());
System.out.println("部门:" + employee.getDepartment());
}
```
在这个main方法中,我们首先创建了一个Employee对象,并使用它的setter方法来设置其属性的值。然后,我们使用其getter方法获取这些属性的值,并使用System.out.println()方法将其输出到控制台上,以检查这些方法是否正常运行。
总之,Employee类是一个通过继承Person类来实现的类,它有两个成员变量salary和department,我们可以使用getter和setter方法来访问它们。为了检查这个新建类中的所有方法,我们可以编写一个主程序来测试它们的正确性。
### 回答3:
首先,我们需要明确继承的概念。子类继承父类的属性和方法,拥有自己的独有属性和方法,并且可以覆盖(override)父类的方法。因此,我们可以定义一个名为employee的类,它继承person类,代码如下:
```
class employee(person):
def __init__(self, name, age, gender, salary, department):
super().__init__(name, age, gender)
self.salary = salary
self.department = department
def get_salary(self):
return self.salary
def set_salary(self, salary):
self.salary = salary
def get_department(self):
return self.department
def set_department(self, department):
self.department = department
```
在这里,我们在子类的构造方法中调用了父类的构造方法,并传入了name、age和gender参数。同时我们新增了salary和department两个属性,并新增了对应的getter和setter方法,以便于获取和设置这两个属性。
为了检查新增类中的所有方法是否正确,我们需要编写主程序。可以编写以下代码:
```
if __name__ == '__main__':
e = employee('Alice', 25, 'Female', 5000, 'IT Department')
print(f'Name: {e.get_name()}, Age: {e.get_age()}, Gender: {e.get_gender()}, '
f'Salary: {e.get_salary()}, Department: {e.get_department()}')
print('Set Salary to 6000')
e.set_salary(6000)
print(f'Name: {e.get_name()}, Age: {e.get_age()}, Gender: {e.get_gender()}, '
f'Salary: {e.get_salary()}, Department: {e.get_department()}')
print('Set Department to HR Department')
e.set_department('HR Department')
print(f'Name: {e.get_name()}, Age: {e.get_age()}, Gender: {e.get_gender()}, '
f'Salary: {e.get_salary()}, Department: {e.get_department()}')
```
运行程序后,将会输出以下结果:
```
Name: Alice, Age: 25, Gender: Female, Salary: 5000, Department: IT Department
Set Salary to 6000
Name: Alice, Age: 25, Gender: Female, Salary: 6000, Department: IT Department
Set Department to HR Department
Name: Alice, Age: 25, Gender: Female, Salary: 6000, Department: HR Department
```
从结果可以看出,我们成功地创建了一个employee类,并且可以正常地获取和设置其中的属性。这证明了我们所创建的类和方法都是正确的。
阅读全文
相关推荐
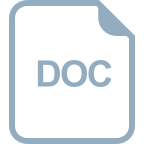
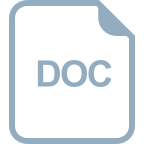
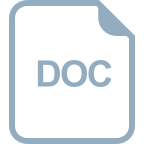












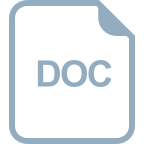
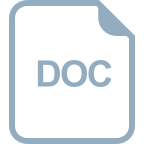