用python的if结构写一个计算个人所得税的案例
时间: 2023-04-07 20:03:43 浏览: 165
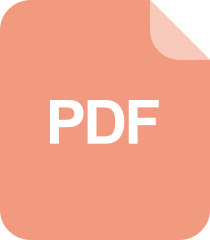
Python实现的个人所得税计算器示例
好的,我可以回答这个问题。以下是一个用 Python 的 if 结构写的计算个人所得税的案例:
income = float(input("请输入您的收入:"))
if income <= 5000:
tax = 0
elif income <= 8000:
tax = (income - 5000) * 0.03
elif income <= 17000:
tax = (income - 8000) * 0.1 + 210
elif income <= 30000:
tax = (income - 17000) * 0.2 + 1410
elif income <= 40000:
tax = (income - 30000) * 0.25 + 2660
elif income <= 60000:
tax = (income - 40000) * 0.3 + 4410
elif income <= 85000:
tax = (income - 60000) * 0.35 + 7160
else:
tax = (income - 85000) * 0.45 + 15160
print("您的个人所得税为:", tax)
请注意,这只是一个简单的计算个人所得税的案例,实际情况可能更加复杂,需要考虑更多的因素。
阅读全文
相关推荐

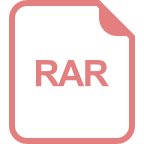
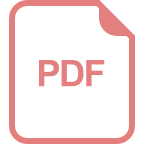
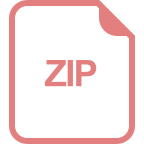
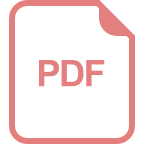
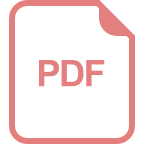
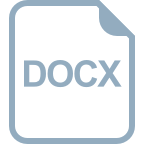
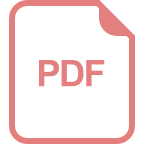
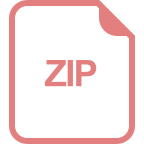
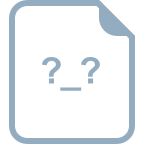
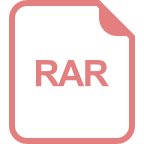
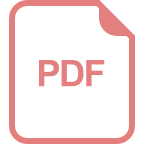
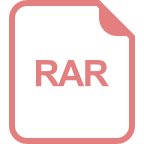