根据上下文,encrypt_data字节数据按Base64编码,得到Base64编码字符串
时间: 2024-04-02 20:32:08 浏览: 72
好的,根据上下文,我们需要将字节数据 `encrypt_data` 进行 Base64 编码,得到一个 Base64 编码的字符串。可以使用 Python 的 `base64` 模块来实现。具体的代码如下:
```python
import base64
# 待编码的字节数据
encrypt_data = b'\xb0\x1a6\x8b\x9d\x86\x08\x19\xe7\xf4\x9c\x1d\xae\x86\xdd'
# 对字节数据进行 Base64 编码
encrypt_text = base64.b64encode(encrypt_data).decode('utf-8')
# 输出 Base64 编码字符串
print(encrypt_text)
```
这里的字节数据 `encrypt_data` 只是一个示例,实际上应该是加密后的字节数据。然后使用 `base64.b64encode()` 方法将字节数据进行 Base64 编码,并使用 `decode()` 方法将其转换成字符串类型。最后使用 `print()` 方法输出 Base64 编码字符串。
需要注意的是,在实际应用中,加密后的字节数据也需要进行保密,不能明文暴露。这里只是为了演示方便,直接使用了一个示例字节数据。
相关问题
根据上下文,使用key和iv对byte_data字节数据做AES/CTR/PKCS5Padding加密,得到加密后字节数据
好的,根据上下文,我们需要使用 AES/CTR/PKCS5Padding 加密算法,使用密钥和 IV 对字节数据 `byte_data` 进行加密。可以使用 Python 中的 `pycryptodome` 库来实现。具体的代码如下:
```python
from Crypto.Cipher import AES
import base64
# 待加密的数据
text = "这是一条测试消息"
byte_data = text.encode('utf-8')
# 加密密钥和 IV
key = b'0123456789abcdef'
iv = b'0123456789abcdef'
# 创建 AES 对象并进行加密
cipher = AES.new(key, AES.MODE_CTR, iv)
encrypt_data = cipher.encrypt(byte_data)
# 将加密后的数据进行 Base64 编码
encrypt_text = base64.b64encode(encrypt_data).decode('utf-8')
# 输出加密后的 Base64 编码字符串
print(encrypt_text)
```
这里首先将待加密的文本数据 `text` 使用 UTF-8 编码转换成字节数据 `byte_data`。然后定义密钥和 IV,创建 AES 对象,使用 `encrypt()` 方法对字节数据进行加密,得到加密后的字节数据并将其存储在 `encrypt_data` 变量中。接着使用 `base64.b64encode()` 方法将加密后的字节数据进行 Base64 编码,并使用 `decode()` 方法将其转换成字符串类型。最后使用 `print()` 方法输出加密后的 Base64 编码字符串。
需要注意的是,在实际应用中,密钥和 IV 需要保密,不能明文写在代码中。这里只是为了演示方便,直接在代码中定义了密钥和 IV。
阅读全文
相关推荐
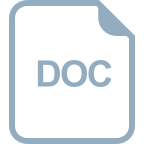
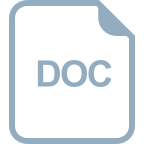
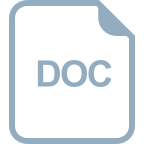
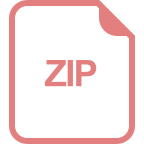
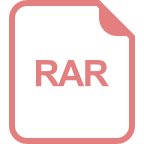
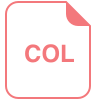
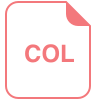
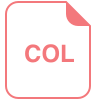
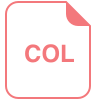
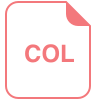
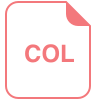
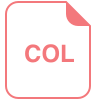
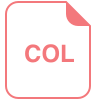
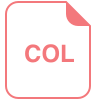
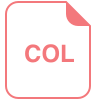
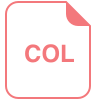
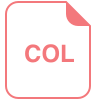
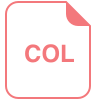