class ChannelAttention(layers.Layer): def __init__(self, in_planes, ratio=32): super(ChannelAttention, self).__init__() self.avg= layers.GlobalAveragePooling2D() self.max= layers.GlobalMaxPooling2D() self.conv1 = layers.Conv2D(in_planes//ratio, kernel_size=1, strides=1, padding='same', kernel_regularizer=regularizers.l2(5e-4), use_bias=True, activation=tf.nn.relu) self.conv2 = layers.Conv2D(in_planes, kernel_size=1, strides=1, padding='same', kernel_regularizer=regularizers.l2(5e-4), use_bias=True) def call(self, inputs): avg = self.avg(inputs) max = self.max(inputs) avg = layers.Reshape((1, 1, avg.shape[1]))(avg) # shape (None, 1, 1 feature) max = layers.Reshape((1, 1, max.shape[1]))(max) # shape (None, 1, 1 feature) avg_out = self.conv2(self.conv1(avg)) max_out = self.conv2(self.conv1(max)) out = avg_out + max_out out = tf.nn.sigmoid(out) return out 每句代码是什么意思
时间: 2024-04-27 13:24:36 浏览: 156
这段代码定义了一个继承自`layers.Layer`的自定义Keras层`ChannelAttention`,用于实现通道注意力机制。具体代码含义如下:
- `def __init__(self, in_planes, ratio=32):` 定义了构造函数,其中`in_planes`表示输入的通道数,`ratio`表示压缩比例,默认为32。
- `super(ChannelAttention, self).__init__()` 调用父类的构造函数。
- `self.avg= layers.GlobalAveragePooling2D()` 定义了全局平均池化层,用于对每个通道的特征图求平均值。
- `self.max= layers.GlobalMaxPooling2D()` 定义了全局最大池化层,用于对每个通道的特征图求最大值。
- `self.conv1 = layers.Conv2D(in_planes//ratio, kernel_size=1, strides=1, padding='same', kernel_regularizer=regularizers.l2(5e-4), use_bias=True, activation=tf.nn.relu)` 定义了第一个卷积层,用于对平均值和最大值进行降维,其中`in_planes//ratio`表示降维后的通道数,`kernel_size=1`表示卷积核的大小为1x1,`padding='same'`表示使用padding方式为“same”,`kernel_regularizer=regularizers.l2(5e-4)`表示使用L2正则化来惩罚大的权重值,防止过拟合,`use_bias=True`表示使用偏置,`activation=tf.nn.relu`表示激活函数为ReLU。
- `self.conv2 = layers.Conv2D(in_planes, kernel_size=1, strides=1, padding='same', kernel_regularizer=regularizers.l2(5e-4), use_bias=True)` 定义了第二个卷积层,用于将降维后的特征图升维回原来的通道数。
- `def call(self, inputs):` 定义了`call`方法,其中`inputs`表示输入的特征图。
- `avg = self.avg(inputs)` 对输入特征图进行全局平均池化。
- `max = self.max(inputs)` 对输入特征图进行全局最大池化。
- `avg = layers.Reshape((1, 1, avg.shape[1]))(avg)` 将平均值的形状变为`(1, 1, feature)`,其中`feature`表示通道数。
- `max = layers.Reshape((1, 1, max.shape[1]))(max)` 将最大值的形状变为`(1, 1, feature)`,其中`feature`表示通道数。
- `avg_out = self.conv2(self.conv1(avg))` 对平均值进行降维和升维操作。
- `max_out = self.conv2(self.conv1(max))` 对最大值进行降维和升维操作。
- `out = avg_out + max_out` 将降维后的平均值和最大值相加。
- `out = tf.nn.sigmoid(out)` 对相加后的结果进行sigmoid激活,得到输出的注意力系数。
- `return out` 返回输出的注意力系数。
阅读全文
相关推荐
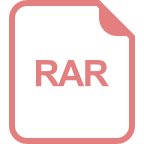
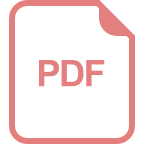
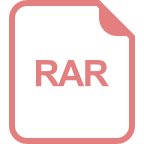






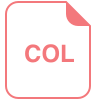
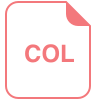

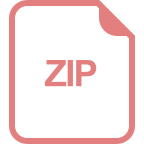
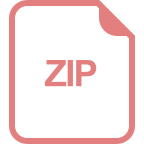
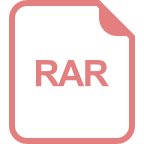
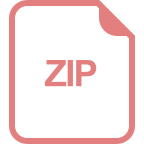
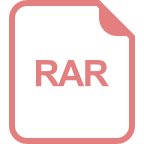
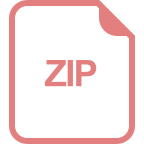