c++中map容器怎么遍历
时间: 2024-05-04 16:18:02 浏览: 8
在 C++ 中有 STL(Standard Template Library)标准模板库,其中包含了很多方便实用的容器,map 就是其中之一。而在 C 语言中,并没有直接提供 map 容器,但是可以使用结构体和链表等数据结构来实现类似的功能。
如果你想遍历 C++ 中的 map 容器,可以使用迭代器来实现,代码示例如下:
```cpp
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> my_map;
my_map["apple"] = 3;
my_map["banana"] = 6;
my_map["orange"] = 9;
for (auto iter = my_map.begin(); iter != my_map.end(); ++iter) {
cout << "key: " << iter->first << ", value: " << iter->second << endl;
}
return 0;
}
```
输出结果为:
```
key: apple, value: 3
key: banana, value: 6
key: orange, value: 9
```
如果你想在 C 语言中实现类似的功能,可以使用结构体和链表来实现,代码示例如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct node {
char key[20];
int value;
struct node* next;
};
void map_put(struct node** map, char* key, int value) {
struct node* new_node = (struct node*)malloc(sizeof(struct node));
strcpy(new_node->key, key);
new_node->value = value;
new_node->next = NULL;
if (*map == NULL) {
*map = new_node;
return;
}
struct node* cur_node = *map;
while (cur_node->next != NULL) {
if (strcmp(cur_node->key, key) == 0) {
cur_node->value = value;
return;
}
cur_node = cur_node->next;
}
if (strcmp(cur_node->key, key) == 0) {
cur_node->value = value;
free(new_node);
return;
}
cur_node->next = new_node;
}
int map_get(struct node* map, char* key) {
struct node* cur_node = map;
while (cur_node != NULL) {
if (strcmp(cur_node->key, key) == 0) {
return cur_node->value;
}
cur_node = cur_node->next;
}
return -1;
}
void map_remove(struct node** map, char* key) {
struct node* cur_node = *map;
struct node* prev_node = NULL;
while (cur_node != NULL) {
if (strcmp(cur_node->key, key) == 0) {
if (prev_node == NULL) {
*map = cur_node->next;
} else {
prev_node->next = cur_node->next;
}
free(cur_node);
return;
}
prev_node = cur_node;
cur_node = cur_node->next;
}
}
void map_clear(struct node** map) {
struct node* cur_node = *map;
while (cur_node != NULL) {
struct node* next_node = cur_node->next;
free(cur_node);
cur_node = next_node;
}
*map = NULL;
}
void map_print(struct node* map) {
struct node* cur_node = map;
while (cur_node != NULL) {
printf("key: %s, value: %d\n", cur_node->key, cur_node->value);
cur_node = cur_node->next;
}
}
int main() {
struct node* my_map = NULL;
map_put(&my_map, "apple", 3);
map_put(&my_map, "banana", 6);
map_put(&my_map, "orange", 9);
map_print(my_map);
map_remove(&my_map, "banana");
printf("\nAfter remove:\n");
map_print(my_map);
map_clear(&my_map);
return 0;
}
```
输出结果为:
```
key: apple, value: 3
key: banana, value: 6
key: orange, value: 9
After remove:
key: apple, value: 3
key: orange, value: 9
```
相关推荐
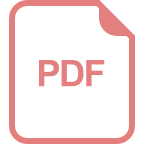
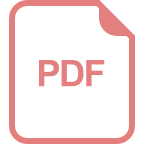
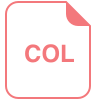
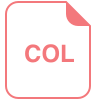
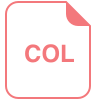
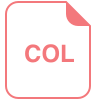










