用Java二、 编写选号程序,在窗体中安排6个标签,每个标签上显示0~9之间的一位数字,每位数字用一个线程控制其变化,单击“停止”则所有标签数字停止变化。
时间: 2024-02-25 16:59:29 浏览: 60
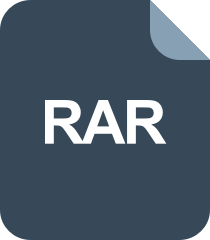
java 标签的制作
下面是一个简单的 Java 代码实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class NumberPicker extends JFrame implements ActionListener {
private JLabel[] labels;
private Thread[] threads;
private boolean running;
public NumberPicker() {
super("Number Picker");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
// 初始化标签和线程
labels = new JLabel[6];
threads = new Thread[6];
for (int i = 0; i < 6; i++) {
labels[i] = new JLabel("0");
add(labels[i]);
threads[i] = new Thread(new NumberPickerThread(i));
threads[i].start();
}
// 添加“停止”按钮
JButton stopButton = new JButton("停止");
stopButton.addActionListener(this);
add(stopButton, BorderLayout.SOUTH);
// 设置窗口大小和可见性
setSize(300, 100);
setResizable(false);
setVisible(true);
}
// 按钮点击事件
public void actionPerformed(ActionEvent event) {
running = false;
}
// 线程类,控制标签的数字变化
private class NumberPickerThread implements Runnable {
private int index;
public NumberPickerThread(int index) {
this.index = index;
}
public void run() {
while (running) {
// 随机生成一个数字并更新标签
int number = (int) (Math.random() * 10);
labels[index].setText(Integer.toString(number));
// 等待一段时间再更新
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public static void main(String[] args) {
new NumberPicker();
}
}
```
在这个程序中,我们创建了一个 `NumberPicker` 类,继承自 `JFrame`,同时实现了 `ActionListener` 接口,用来监听“停止”按钮的点击事件。在构造函数中,我们初始化了6个标签和对应的线程,并且在窗口可见时启动线程。每个线程都会随机生成一个数字并更新对应的标签,然后等待一段时间再更新。当用户点击“停止”按钮时,我们将 `running` 变量设置为 `false`,这样所有线程都会停止更新标签。
阅读全文
相关推荐
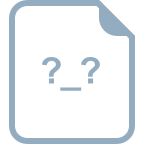



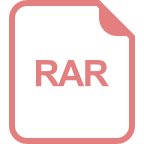
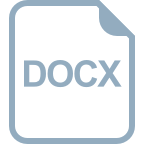
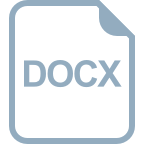
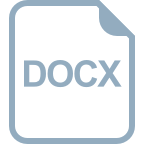
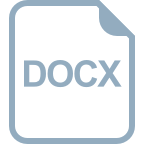
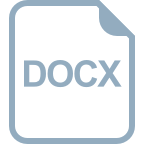
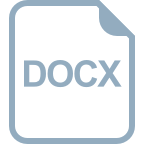
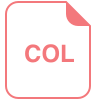
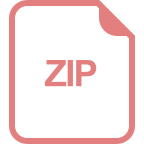
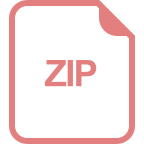
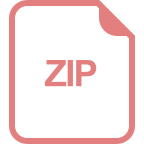