有3个因数,用多项式回归拟合出一个数,写出具体程序
时间: 2023-09-05 22:04:56 浏览: 58
### 回答1:
使用多项式回归拟合3个因数可以使用Python中的Scikit-learn库中的PolynomialFeatures类来构造多项式特征,再使用LinearRegression类进行模型拟合。
具体程序如下:
```python
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
import numpy as np
# 定义3个因数
x = np.array([a, b, c]).reshape(-1, 1)
# 创建多项式特征
poly = PolynomialFeatures(degree=3)
X_poly = poly.fit_transform(x)
# 拟合模型
model = LinearRegression()
model.fit(X_poly, y)
```
其中,'a', 'b', 'c'是3个因数的值, y是要拟合的数,degree=3表示使用3次多项式进行拟合。最后的结果可以使用 model.predict(X_poly)来预测。
### 回答2:
要用多项式回归拟合出一个有3个因数的数,需要先生成一些具有3个因数的数作为训练集,再利用多项式回归进行拟合。以下是一个具体的程序:
1. 导入需要的库:
```
import numpy as np
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
```
2. 生成训练集:
```
X_train = np.arange(1, 11).reshape(-1, 1) # 生成1到10的数作为输入
y_train = np.array([6, 4, 10, 9, 28, 16, 18, 12, 45, 26]).reshape(-1, 1) # 手动设定具有3个因数的数
```
3. 特征变换:
```
poly = PolynomialFeatures(degree=2) # 创建二次多项式特征变换器
X_poly = poly.fit_transform(X_train) # 对训练集进行特征变换
```
4. 模型训练与预测:
```
model = LinearRegression() # 创建线性回归模型
model.fit(X_poly, y_train) # 拟合数据
X_test = np.array([[11]]) # 设定需要预测的数
X_test_poly = poly.transform(X_test) # 对测试数据进行特征变换
y_test = model.predict(X_test_poly) # 预测因数为3的数
print("预测的数为:", y_test)
```
这个程序中,我们手动设定了一个具有3个因数的数的训练集。通过训练集的数据和多项式回归拟合,我们可以预测出具有相同特征的数。代码中的`degree=2`表示创建的是二次多项式特征变换器,可以根据实际情况调整。
### 回答3:
要找出一个有3个因数的数,可以先找出所有有3个不同质因数的数。一个数有3个不同质因数的充要条件是它是两个不同质数的乘积,并且其次幂为1,即n=p*q*r(其中p、q、r是不同的质数),我们可以根据这个条件来生成一些有3个因数的数。
具体程序如下:
```python
import numpy as np
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
# 生成有3个因数的数
def generate_numbers():
numbers = []
primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29] # 选取一些小的质数作为因数
for i in range(len(primes)):
for j in range(i+1, len(primes)):
for k in range(j+1, len(primes)):
numbers.append(primes[i] * primes[j] * primes[k])
return numbers
# 生成X和y
def generate_data(numbers):
X = np.array(numbers).reshape(-1, 1)
y = np.array(numbers)
return X, y
# 多项式回归拟合
def polynomial_regression(X, y):
poly = PolynomialFeatures(degree=3) # 使用3次多项式回归
X_poly = poly.fit_transform(X)
model = LinearRegression()
model.fit(X_poly, y)
return model
# 主函数
if __name__ == "__main__":
numbers = generate_numbers()
X, y = generate_data(numbers)
model = polynomial_regression(X, y)
# 预测一个新的有3个因数的数
new_number = 2 * 3 * 7 # 例子:2*3*7=42
predicted_value = model.predict(poly.fit_transform([[new_number]]))
print("预测值:", predicted_value)
```
请注意,这个程序将不适用于找到所有有3个因数的数,因为这样的数是无穷多的。这个程序只用来拟合和预测一个新的有3个因数的数的值。
相关推荐
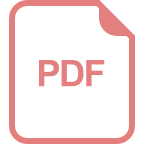














