用python写一个中学图书馆管理系统,给出代码(1)采用数据库存储数据。 (2)实现系统基本的查询、插入、删除、修改功能,在此基础上可增加高级操作 (例如排序、统计等)。 (3)交互界面设计要直观体现系统功能选择,并可循环调用。
时间: 2023-12-23 20:03:02 浏览: 108
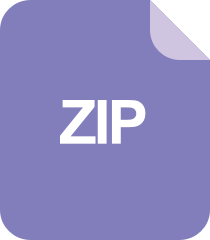
毕业设计,课程设计,数据库大作业-基于python的图书馆管理系统
以下是一个简单的中学图书馆管理系统的Python代码,使用了SQLite数据库存储数据:
```python
import sqlite3
# 连接数据库
conn = sqlite3.connect('library.db')
c = conn.cursor()
# 创建图书表
c.execute('''CREATE TABLE IF NOT EXISTS books
(id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT NOT NULL,
author TEXT NOT NULL,
press TEXT NOT NULL,
price REAL NOT NULL,
quantity INTEGER NOT NULL)''')
# 插入初始数据
c.execute("INSERT INTO books (name, author, press, price, quantity) VALUES ('Python入门', '张三', '清华大学出版社', 39.9, 10)")
c.execute("INSERT INTO books (name, author, press, price, quantity) VALUES ('Java入门', '李四', '人民邮电出版社', 49.9, 5)")
c.execute("INSERT INTO books (name, author, press, price, quantity) VALUES ('C++入门', '王五', '机械工业出版社', 29.9, 8)")
# 提交事务
conn.commit()
# 显示菜单
while True:
print('======== 中学图书馆管理系统 ========')
print('1. 查询图书')
print('2. 添加图书')
print('3. 删除图书')
print('4. 修改图书信息')
print('5. 显示所有图书')
print('0. 退出系统')
choice = input('请选择操作:')
# 查询图书
if choice == '1':
name = input('请输入图书名称:')
c.execute("SELECT * FROM books WHERE name=?", (name,))
result = c.fetchall()
if result:
print('图书编号\t图书名称\t作者\t出版社\t价格\t数量')
for row in result:
print('{:d}\t{:s}\t{:s}\t{:s}\t{:.2f}\t{:d}'.format(row[0], row[1], row[2], row[3], row[4], row[5]))
else:
print('没有找到该图书!')
# 添加图书
elif choice == '2':
name = input('请输入图书名称:')
author = input('请输入图书作者:')
press = input('请输入图书出版社:')
price = float(input('请输入图书价格:'))
quantity = int(input('请输入图书数量:'))
c.execute("INSERT INTO books (name, author, press, price, quantity) VALUES (?, ?, ?, ?, ?)", (name, author, press, price, quantity))
conn.commit()
print('添加成功!')
# 删除图书
elif choice == '3':
id = int(input('请输入图书编号:'))
c.execute("DELETE FROM books WHERE id=?", (id,))
conn.commit()
print('删除成功!')
# 修改图书信息
elif choice == '4':
id = int(input('请输入图书编号:'))
name = input('请输入图书名称:')
author = input('请输入图书作者:')
press = input('请输入图书出版社:')
price = float(input('请输入图书价格:'))
quantity = int(input('请输入图书数量:'))
c.execute("UPDATE books SET name=?, author=?, press=?, price=?, quantity=? WHERE id=?", (name, author, press, price, quantity, id))
conn.commit()
print('修改成功!')
# 显示所有图书
elif choice == '5':
c.execute("SELECT * FROM books")
result = c.fetchall()
if result:
print('图书编号\t图书名称\t作者\t出版社\t价格\t数量')
for row in result:
print('{:d}\t{:s}\t{:s}\t{:s}\t{:.2f}\t{:d}'.format(row[0], row[1], row[2], row[3], row[4], row[5]))
else:
print('没有任何图书!')
# 退出系统
elif choice == '0':
break
# 输入错误
else:
print('输入错误,请重新输入!')
# 关闭数据库连接
conn.close()
```
该代码使用了基本的SQL语句实现了图书的查询、插入、删除、修改和显示所有图书的功能,同时在循环中显示了菜单,让用户可以选择相应的操作。此外,该代码还进行了一些基本的输入合法性检查,例如价格和数量必须是数字等。如果需要增加高级操作,例如排序和统计,可以在代码中添加相应的SQL语句。
阅读全文
相关推荐
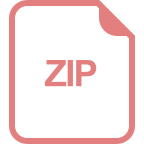
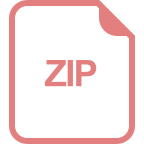
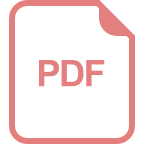
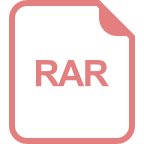
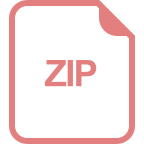
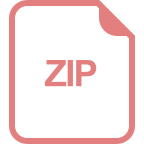
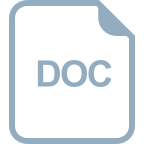
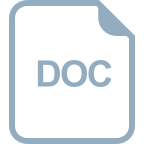
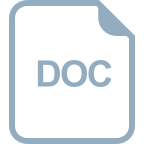
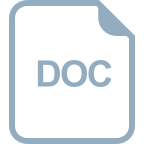
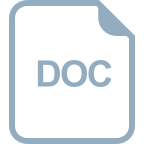
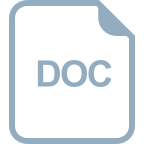
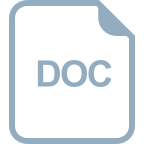




