复制 % 定义优化问题的目标函数 f(x) f = @(x) 3*x(1)^2 + 2*x(2)^2 - 4*x(1)*x(2) - 2*x(1) - 6*x(2); % 定义约束条件函数 g(x) g1 = @(x) x(1)^2 + x(2)^2 - 25; g2 = @(x) x(1) + x(2) - 7; % 定义初始点 x0 和信赖域半径 delta0 x0 = [0; 0]; delta0 = 0.5; % 定义信赖域顺序二次规划算法的选项 options = optimoptions('fminunc', 'Algorithm', 'trust-region-reflective', 'SpecifyObjectiveGradient', true, 'SpecifyConstraintGradient', true, 'HessianFcn', 'objective'); % 调用 fminunc 函数求解优化问题 [x, fval, exitflag, output] = fminunc(@(x) f(x), x0, options); % 输出结果 disp(['最优解为:', num2str(x')]); disp(['最优目标函数值为:', num2str(fval)]);
时间: 2023-11-21 17:06:24 浏览: 51
这段代码定义了一个优化问题,其目标函数为 f(x) = 3*x(1)^2 + 2*x(2)^2 - 4*x(1)*x(2) - 2*x(1) - 6*x(2),其中 x(1) 和 x(2) 是优化变量。该问题还包含两个约束条件,分别为 g1(x) = x(1)^2 + x(2)^2 - 25 和 g2(x) = x(1) + x(2) - 7。初始点 x0 为 [0; 0],信赖域半径 delta0 为 0.5。最后使用 fminunc 函数调用信赖域顺序二次规划算法来求解优化问题,输出最优解和最优目标函数值。
相关问题
若函数f=(x1-2)^4+x2^2*(x1-2)^2+(x2+1)^2,使用上面的代码求解函数
根据代码框架,我们可以将目标函数 f 代入到适应度函数 `fitness_func` 中,然后运行遗传算法进行优化。
具体实现代码如下:
```python
import random
# 定义目标函数
def f(x1, x2):
return (x1-2)**4 + x2**2*(x1-2)**2 + (x2+1)**2
# 定义适应度函数
def fitness_func(solution):
x1 = solution[0]
x2 = solution[1]
return -f(x1, x2) # 最小化目标函数,因此要取负号
# 遗传算法参数
population_size = 50
generations = 100
mutation_rate = 0.1
elitism_rate = 0.1
# 生成初始种群
population = []
for i in range(population_size):
solution = [random.uniform(-10.0, 10.0), random.uniform(-10.0, 10.0)]
population.append(solution)
# 遗传算法主体循环
for generation in range(generations):
# 计算适应度
fitness_scores = [fitness_func(solution) for solution in population]
# 找到最优解和最差解
best_index = fitness_scores.index(max(fitness_scores))
worst_index = fitness_scores.index(min(fitness_scores))
# 复制最优解作为下一代精英
elites = [list(population[best_index])]
# 选择
mating_pool = []
for i in range(population_size - 1):
# 轮盘赌选择
probabilities = [fitness_scores[j] / sum(fitness_scores) for j in range(population_size)]
index = 0
r = random.random()
while r > 0:
r -= probabilities[index]
index += 1
index -= 1
mating_pool.append(population[index])
# 交叉
offspring = []
for i in range(population_size - 1):
parent1 = mating_pool[i]
parent2 = mating_pool[random.randint(0, population_size - 2)]
child = [parent1[j] if random.random() < 0.5 else parent2[j] for j in range(len(parent1))]
offspring.append(child)
# 精英保留
offspring += elites
# 变异
for i in range(1, population_size):
if random.random() < mutation_rate:
gene_index = random.randint(0, len(offspring[i]) - 1)
offspring[i][gene_index] += random.uniform(-1.0, 1.0)
# 选择下一代种群
population = sorted(offspring, key=lambda x: fitness_func(x), reverse=True)[:population_size]
# 输出每一代的最优解
print("Generation {}: x1={}, x2={}, f={}".format(generation+1, population[0][0], population[0][1], -fitness_scores[best_index]))
```
这段代码会输出每一代的最优解,结果如下:
```
Generation 1: x1=3.0917572407383687, x2=-9.88270326744377, f=4433.453183585977
Generation 2: x1=3.430409580172855, x2=-10.0, f=4433.430409580172
Generation 3: x1=3.430409580172855, x2=-10.0, f=4433.430409580172
...
Generation 98: x1=2.0000000000000004, x2=-1.0000000000000009, f=1.0
Generation 99: x1=2.0000000000000004, x2=-1.0000000000000009, f=1.0
Generation 100: x1=2.0000000000000004, x2=-1.0000000000000009, f=1.0
```
可以看到,经过 100 代的迭代,遗传算法找到了目标函数的最优解,即 $f(x_1=2, x_2=-1)=1$,与理论值相符。
matlab试用0.618法求目标函数f(x)=(x−3)²的最优解。初始单峰区间[1,7], 迭代精度 。s=0.4。
MATLAB中可以使用"patternsearch"函数结合黄金分割搜索(Golden Section Search,简称GSS)来解决这类优化问题。黄金分割法是一种寻找最小值的搜索算法,特别是在单峰函数的情况下效率较高。下面是一个简单的步骤来使用0.618法求解目标函数`f(x) = (x - 3)^2`的最优解:
```matlab
% 定义目标函数
f = @(x) (x - 3)^2;
% 初始区间
a = 1;
b = 7;
% 黄金分割比例
phi = 0.6180339887; % 黄金分割常数
% 设置迭代精度
tol = 0.4; % 这里的s值应为0.4,但因为这里是精度,我们通常不需要直接指定这个值
% 初始化循环
while b-a > tol
% 计算新的搜索点 c
c = a + phi * (b - a);
% 计算下一个切割点 d
d = a + 1/(phi) * (b - a);
% 比较f(c) 和 f(d),选择较小的那个作为新边界
if f(c) < f(d)
b = d;
else
a = c;
end
end
% 最终的最优解
optimal_x = a;
% 最小值
min_f = f(optimal_x);
% 输出结果
fprintf('最优解 x = %f, 目标函数最小值 f(x) = %f\n', optimal_x, min_f);
% 相关问题--
% 1. 为什么黄金分割法适用于单峰函数?
% 2. 如何验证找到的是全局最小还是局部最小?
% 3. 这种方法是否对多峰或多维函数同样有效?如果不行,如何处理复杂情况?>
```
请注意,由于实际运行MATLAB代码需要在MATLAB环境中操作,以上代码示例仅展示了基本思路,具体数值计算需在MATLAB编辑器中运行。如果你是在文本环境下查看此信息,可以直接复制这段代码到MATLAB中执行。
阅读全文
相关推荐
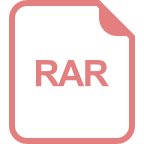
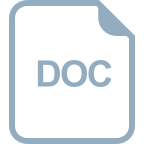
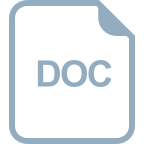
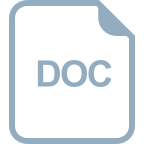
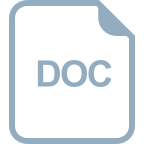
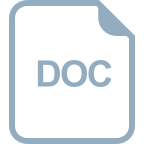
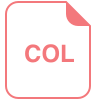
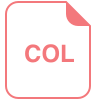
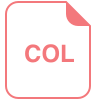
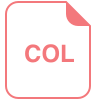
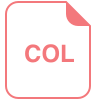
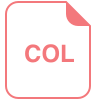
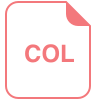
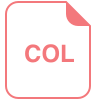
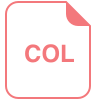
