def forward(self, x): x = torch.relu(self.conv1(x)) self.conv1_l1norm.data = torch.sum(torch.abs(self.conv1.weight.data), dim=(1, 2, 3)) x = torch.relu(self.conv2(x)) self.conv2_l1norm.data = torch.sum(torch.abs(self.conv2.weight.data), dim=(1, 2, 3)) x = x.view(x.size(0), -1) x = self.fc(x) return x
时间: 2023-10-20 09:45:12 浏览: 60
这段代码是一个神经网络模型的前向传播。假设这个模型有两个卷层(`conv1`和`conv2`一个全连接层(`fc`)。以下是代码的解释:
1. `x = torch.relu(self.conv1(x))`将输入数据 `x`通过第一个卷积层 `conv1`,然后应用 ReLU 激活函数。ReLU 函数将负数置为零,保留正数。
2. `self.conv1_l1norm.data = torch.sum(torch.abs(self.conv1.weight.data), dim=(1, 2, 3))`:计算第一个卷积层权重 `conv1.weight` 的 L1 范数,并将结果保存在 `conv1_l1norm` 变量中。L1 范数是指向量中所有元素绝对值的和。
3. `x = torch.relu(self.conv2(x))`:将第一步的输出 `x` 通过第二个卷积层 `conv2`,然后应用 ReLU 激活函数。
4. `self.conv2_l1norm.data = torch.sum(torch.abs(self.conv2.weight.data), dim=(1, 2, 3))`:计算第二个卷积层权重 `conv2.weight` 的 L1 范数,并将结果保存在 `conv2_l1norm` 变量中。
5. `x = x.view(x.size(0), -1)`:将最后一个卷积层的输出展平为一维向量,以适应全连接层的输入要求。
6. `x = self.fc(x)`:将展平后的向量 `x` 输入到全连接层 `fc` 中,进行最后的线性变换。
7. 返回最终输出 `x`。
相关推荐
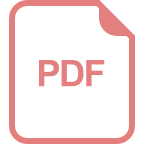
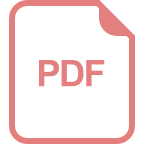
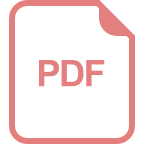














