def conv_dw(filter_in, filter_out, stride = 1):是什么意思
时间: 2024-05-22 19:11:35 浏览: 123
这是一个深度可分离卷积(depthwise separable convolution)的函数定义,常用于卷积神经网络的设计中。
深度可分离卷积是一种卷积操作,它将标准卷积分成两个步骤:深度卷积和逐点卷积。深度卷积只卷积输入的每个通道内的每个滤波器,而逐点卷积是在深度卷积之后应用的,用于将每个通道的输出与其他通道的输出混合。
这个函数定义中,`filter_in`表示输入图像的通道数,`filter_out`表示输出图像的通道数,`stride`表示卷积步长。
相关问题
请分析这段代码class GhostBottleneck(nn.Module): """ Ghost bottleneck w/ optional SE""" def __init__(self, in_chs, mid_chs, out_chs, dw_kernel_size=3, stride=1, act_layer=nn.ReLU, se_ratio=0.): super(GhostBottleneck, self).__init__() has_se = se_ratio is not None and se_ratio > 0. self.stride = stride # Point-wise expansion self.ghost1 = GhostModule(in_chs, mid_chs, relu=True) # Depth-wise convolution if self.stride > 1: self.conv_dw = nn.Conv2d(mid_chs, mid_chs, dw_kernel_size, stride=stride, padding=(dw_kernel_size - 1) // 2, groups=mid_chs, bias=False) self.bn_dw = nn.BatchNorm2d(mid_chs) # Squeeze-and-excitation if has_se: self.se = SqueezeExcite(mid_chs, se_ratio=se_ratio) else: self.se = None # Point-wise linear projection self.ghost2 = GhostModule(mid_chs, out_chs, relu=False) # shortcut if (in_chs == out_chs and self.stride == 1): self.shortcut = nn.Sequential() else: self.shortcut = nn.Sequential( nn.Conv2d(in_chs, in_chs, dw_kernel_size, stride=stride, padding=(dw_kernel_size - 1) // 2, groups=in_chs, bias=False), nn.BatchNorm2d(in_chs), nn.Conv2d(in_chs, out_chs, 1, stride=1, padding=0, bias=False), nn.BatchNorm2d(out_chs), ) def forward(self, x): residual = x # 1st ghost bottleneck x = self.ghost1(x) # Depth-wise convolution if self.stride > 1: x = self.conv_dw(x) x = self.bn_dw(x) # Squeeze-and-excitation if self.se is not None: x = self.se(x) # 2nd ghost bottleneck x = self.ghost2(x) x += self.shortcut(residual) return x
这段代码定义了一个名为GhostBottleneck的类,继承自nn.Module。该类实现了一个带有可选Squeeze-and-excitation (SE)的Ghost bottleneck。
在初始化方法中,它接受一些参数,包括输入通道数(in_chs)、中间通道数(mid_chs)、输出通道数(out_chs)、深度卷积核大小(dw_kernel_size)、步长(stride)、激活函数(act_layer)和SE比率(se_ratio)。它首先判断是否需要SE操作,并保存步长。然后,它定义了以下组件:
- ghost1: 一个GhostModule,用于进行点卷积扩展,将输入通道数扩展到中间通道数。
- conv_dw和bn_dw: 如果步长大于1,则定义了一个深度卷积层和对应的批归一化层,用于进行深度卷积操作。
- se: 如果需要SE操作,则定义了一个SqueezeExcite模块,用于进行Squeeze-and-excitation操作。
- ghost2: 一个GhostModule,用于将中间通道数缩减到输出通道数。
- shortcut: 根据输入通道数和输出通道数以及步长的不同情况,定义了不同的shortcut结构。如果输入通道数等于输出通道数且步长为1,则shortcut为空;否则,shortcut由一系列卷积层和批归一化层组成。
在前向传播方法中,首先保存输入的残差,然后按照以下顺序进行操作:
- 使用ghost1进行第一个ghost bottleneck操作,将输入x转换为中间特征x。
- 如果步长大于1,则使用conv_dw和bn_dw进行深度卷积操作。
- 如果需要SE操作,则使用se进行Squeeze-and-excitation操作。
- 使用ghost2进行第二个ghost bottleneck操作,将中间特征x转换为输出特征x。
- 将残差与shortcut结果相加得到最终输出x。
该GhostBottleneck类实现了一种特殊的残差块结构,通过使用GhostModule和深度卷积、SE等操作,实现了通道数的扩展和缩减,并在残差连接中处理了不同通道数和步长的情况。这种结构常用于一些深度学习模型中的卷积层。
class Net(nn.Module): def __init__(self): super(Net, self).__init__() self.conv1 = nn.Conv1d(in_channels=1, out_channels=64, kernel_size=32, stride=8, padding=12) self.pool1 = nn.MaxPool1d(kernel_size=2, stride=2) self.BN = nn.BatchNorm1d(num_features=64) self.conv3_1 = nn.Conv1d(in_channels=64, out_channels=64, kernel_size=3, stride=1, padding=1) self.pool3_1 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv3_2 = nn.Conv1d(in_channels=64, out_channels=128, kernel_size=3, stride=1, padding=1) self.pool3_2 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv3_3 = nn.Conv1d(in_channels=128, out_channels=256, kernel_size=3, stride=1, padding=1) self.pool3_3 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv5_1 = nn.Conv1d(in_channels=64, out_channels=64, kernel_size=5, stride=1, padding=2) self.pool5_1 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv5_2 = nn.Conv1d(in_channels=64, out_channels=128, kernel_size=5, stride=1, padding=2) self.pool5_2 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv5_3 = nn.Conv1d(in_channels=128, out_channels=256, kernel_size=5, stride=1, padding=2) self.pool5_3 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv7_1 = nn.Conv1d(in_channels=64, out_channels=64, kernel_size=7, stride=1, padding=3) self.pool7_1 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv7_2 = nn.Conv1d(in_channels=64, out_channels=128, kernel_size=7, stride=1, padding=3) self.pool7_2 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv7_3 = nn.Conv1d(in_channels=128, out_channels=256, kernel_size=7, stride=1, padding=3) self.pool7_3 = nn.MaxPool1d(kernel_size=2, stride=2) self.pool2 = nn.MaxPool1d(kernel_size=8, stride=1) self.fc = nn.Linear(in_features=256 * 3, out_features=4) ##这里的256*3是计算出来的 self.softmax = nn.Softmax(),解释各部分的作用和参数选择
这段代码定义了一个名为Net的类,继承了nn.Module类。在初始化函数中,它定义了多个一维卷积层(nn.Conv1d)、最大池化层(nn.MaxPool1d)、BN层(nn.BatchNorm1d)和全连接层(nn.Linear)。这些层用于构建神经网络模型。其中,卷积层、池化层和BN层用于特征提取,全连接层用于分类。此模型的输入是一个通道的一维数据,输出是四个类别的概率分布。
阅读全文
相关推荐
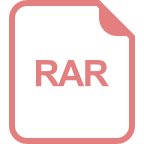
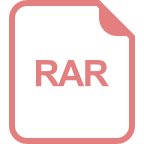
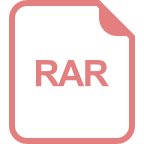











