用python做一个五子棋游戏
时间: 2023-10-08 11:06:57 浏览: 38
好的,下面是一个简单的五子棋游戏的Python代码。这个游戏采用了Pygame库来实现界面和交互。
首先,需要安装Pygame库,可以使用以下命令安装:
```
pip install pygame
```
然后,创建一个名为"five_in_a_row.py"的Python文件,并复制以下代码:
```python
import pygame
import sys
# 初始化Pygame
pygame.init()
# 设置窗口尺寸
WINDOW_SIZE = (640, 640)
screen = pygame.display.set_mode(WINDOW_SIZE)
# 设置标题
pygame.display.set_caption("五子棋")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
# 定义棋盘大小和格子大小
BOARD_SIZE = 15
CELL_SIZE = 40
# 计算棋盘和界面的大小
BOARD_WIDTH = BOARD_SIZE * CELL_SIZE
BOARD_HEIGHT = BOARD_SIZE * CELL_SIZE
SCREEN_WIDTH = BOARD_WIDTH + CELL_SIZE * 2
SCREEN_HEIGHT = BOARD_HEIGHT + CELL_SIZE * 2
# 加载棋子图片
BLACK_IMAGE = pygame.image.load("black.png")
BLACK_IMAGE = pygame.transform.scale(BLACK_IMAGE, (CELL_SIZE, CELL_SIZE))
WHITE_IMAGE = pygame.image.load("white.png")
WHITE_IMAGE = pygame.transform.scale(WHITE_IMAGE, (CELL_SIZE, CELL_SIZE))
# 创建棋盘
board = [[0 for i in range(BOARD_SIZE)] for j in range(BOARD_SIZE)]
# 定义当前落子的颜色
current_player = 1
# 画棋盘
def draw_board():
screen.fill(GRAY)
for i in range(BOARD_SIZE):
pygame.draw.line(screen, BLACK, (CELL_SIZE, CELL_SIZE + i * CELL_SIZE), (BOARD_WIDTH + CELL_SIZE, CELL_SIZE + i * CELL_SIZE))
pygame.draw.line(screen, BLACK, (CELL_SIZE + i * CELL_SIZE, CELL_SIZE), (CELL_SIZE + i * CELL_SIZE, BOARD_HEIGHT + CELL_SIZE))
# 画棋子
def draw_piece(row, col):
x = CELL_SIZE + col * CELL_SIZE
y = CELL_SIZE + row * CELL_SIZE
if board[row][col] == 1:
screen.blit(BLACK_IMAGE, (x, y))
elif board[row][col] == 2:
screen.blit(WHITE_IMAGE, (x, y))
# 判断是否胜利
def check_win(row, col):
# 水平方向
count = 1
for i in range(1, 5):
if col - i < 0 or board[row][col-i] != current_player:
break
count += 1
for i in range(1, 5):
if col + i >= BOARD_SIZE or board[row][col+i] != current_player:
break
count += 1
if count >= 5:
return True
# 垂直方向
count = 1
for i in range(1, 5):
if row - i < 0 or board[row-i][col] != current_player:
break
count += 1
for i in range(1, 5):
if row + i >= BOARD_SIZE or board[row+i][col] != current_player:
break
count += 1
if count >= 5:
return True
# 左上到右下方向
count = 1
for i in range(1, 5):
if row - i < 0 or col - i < 0 or board[row-i][col-i] != current_player:
break
count += 1
for i in range(1, 5):
if row + i >= BOARD_SIZE or col + i >= BOARD_SIZE or board[row+i][col+i] != current_player:
break
count += 1
if count >= 5:
return True
# 左下到右上方向
count = 1
for i in range(1, 5):
if row + i >= BOARD_SIZE or col - i < 0 or board[row+i][col-i] != current_player:
break
count += 1
for i in range(1, 5):
if row - i < 0 or col + i >= BOARD_SIZE or board[row-i][col+i] != current_player:
break
count += 1
if count >= 5:
return True
return False
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
if event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
x, y = event.pos
if CELL_SIZE <= x <= BOARD_WIDTH + CELL_SIZE and CELL_SIZE <= y <= BOARD_HEIGHT + CELL_SIZE:
col = (x - CELL_SIZE) // CELL_SIZE
row = (y - CELL_SIZE) // CELL_SIZE
if board[row][col] == 0:
board[row][col] = current_player
draw_piece(row, col)
if check_win(row, col):
print("player", current_player, "wins!")
pygame.time.delay(2000)
sys.exit()
current_player = 3 - current_player
# 画界面
draw_board()
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] != 0:
draw_piece(i, j)
pygame.display.update()
```
在同一目录下创建两个名为"black.png"和"white.png"的PNG图片,代表黑子和白子。
运行代码后,将会出现一个五子棋的游戏界面。玩家可以使用鼠标点击棋盘上的格子来选择落子位置,程序会自动判断是否存在五子连珠的情况。
注意:这个代码只是一个简单的五子棋游戏实现,还有很多可以改进的地方。
相关推荐
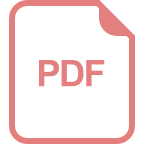
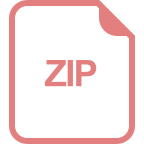











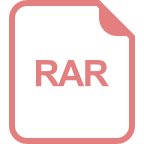
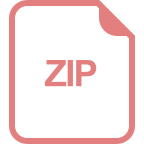
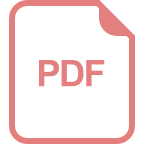
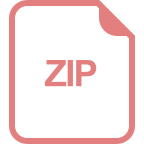