用cv::FileStorage读取文件的代码的所有写法
时间: 2024-03-21 09:42:44 浏览: 30
以下是使用cv::FileStorage读取不同文件格式的示例代码:
1. 读取XML文件:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
FileStorage fs("example.xml", FileStorage::READ);
if (!fs.isOpened())
{
cerr << "Failed to open file!" << endl;
return -1;
}
// 读取数据
int num;
string str;
vector<float> vec;
fs["number"] >> num;
fs["string"] >> str;
fs["vector"] >> vec;
// 输出数据
cout << "number: " << num << endl;
cout << "string: " << str << endl;
cout << "vector: ";
for (size_t i = 0; i < vec.size(); i++)
{
cout << vec[i] << " ";
}
cout << endl;
fs.release();
return 0;
}
```
2. 读取YAML文件:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
FileStorage fs("example.yaml", FileStorage::READ);
if (!fs.isOpened())
{
cerr << "Failed to open file!" << endl;
return -1;
}
// 读取数据
int num;
string str;
vector<float> vec;
fs["number"] >> num;
fs["string"] >> str;
fs["vector"] >> vec;
// 输出数据
cout << "number: " << num << endl;
cout << "string: " << str << endl;
cout << "vector: ";
for (size_t i = 0; i < vec.size(); i++)
{
cout << vec[i] << " ";
}
cout << endl;
fs.release();
return 0;
}
```
3. 读取JSON文件:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
FileStorage fs("example.json", FileStorage::READ);
if (!fs.isOpened())
{
cerr << "Failed to open file!" << endl;
return -1;
}
// 读取数据
int num;
string str;
vector<float> vec;
fs["number"] >> num;
fs["string"] >> str;
fs["vector"] >> vec;
// 输出数据
cout << "number: " << num << endl;
cout << "string: " << str << endl;
cout << "vector: ";
for (size_t i = 0; i < vec.size(); i++)
{
cout << vec[i] << " ";
}
cout << endl;
fs.release();
return 0;
}
```
在以上代码中,我们通过不同的文件扩展名来指定不同格式的文件,并在读取完成后调用`release()`函数以释放内存并关闭文件。
相关推荐
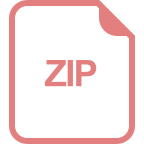















