用C++编写程序,题目描述 无向连通图的广度遍历序列和深度遍历序列的输出。 输入描述 顶点个数,边的条数,边的信息(每条边只输入一次)。 输出描述 从V0出发的广度遍历序列和深度遍历序列 样例输入 9 10 0 1 0 3 0 4 1 2 1 4 2 5 3 6 4 6 6 7 7 8 样例输出 ABDECGFHI ABCFEGHID
时间: 2023-12-06 12:44:32 浏览: 71
好的,我明白了,以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100 // 图的最大顶点数
#define MAX_QUEUE_SIZE 100 // 队列的最大容量
typedef struct {
int vertexes[MAX_VERTEX_NUM]; // 存储顶点信息
int edges[MAX_VERTEX_NUM][MAX_VERTEX_NUM]; // 存储边信息
int vertex_num, edge_num; // 记录顶点数和边数
} Graph;
typedef struct {
int data[MAX_QUEUE_SIZE];
int front, rear;
} Queue;
void init_queue(Queue *queue) {
queue->front = queue->rear = 0;
}
int is_empty(Queue *queue) {
return queue->front == queue->rear;
}
int is_full(Queue *queue) {
return (queue->rear + 1) % MAX_QUEUE_SIZE == queue->front;
}
int enqueue(Queue *queue, int x) {
if (is_full(queue)) {
return 0;
} else {
queue->data[queue->rear] = x;
queue->rear = (queue->rear + 1) % MAX_QUEUE_SIZE;
return 1;
}
}
int dequeue(Queue *queue, int *x) {
if (is_empty(queue)) {
return 0;
} else {
*x = queue->data[queue->front];
queue->front = (queue->front + 1) % MAX_QUEUE_SIZE;
return 1;
}
}
void depth_first_search(Graph *graph, int visited[], int vertex) {
printf("%c", 'A' + vertex);
visited[vertex] = 1;
for (int i = 0; i < graph->vertex_num; i++) {
if (graph->edges[vertex][i] == 1 && !visited[i]) {
depth_first_search(graph, visited, i);
}
}
}
void breadth_first_search(Graph *graph, int visited[], int vertex) {
Queue queue;
init_queue(&queue);
enqueue(&queue, vertex);
visited[vertex] = 1;
while (!is_empty(&queue)) {
int current_vertex;
dequeue(&queue, ¤t_vertex);
printf("%c", 'A' + current_vertex);
for (int i = 0; i < graph->vertex_num; i++) {
if (graph->edges[current_vertex][i] == 1 && !visited[i]) {
enqueue(&queue, i);
visited[i] = 1;
}
}
}
}
int main() {
Graph graph;
int visited[MAX_VERTEX_NUM] = {0};
scanf("%d%d", &graph.vertex_num, &graph.edge_num);
for (int i = 0; i < graph.vertex_num; i++) {
graph.vertexes[i] = i;
}
for (int i = 0; i < graph.edge_num; i++) {
int start, end;
scanf("%d%d", &start, &end);
graph.edges[start][end] = graph.edges[end][start] = 1;
}
depth_first_search(&graph, visited, 0); // 从V0开始深度优先遍历
printf("\n");
for (int i = 0; i < graph.vertex_num; i++) {
visited[i] = 0;
}
breadth_first_search(&graph, visited, 0); // 从V0开始广度优先遍历
return 0;
}
```
该程序使用邻接矩阵存储图,同时实现了深度优先遍历和广度优先遍历。其中深度优先遍历是通过递归实现的,广度优先遍历是通过队列实现的。
阅读全文
相关推荐
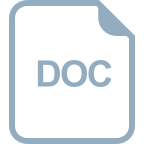
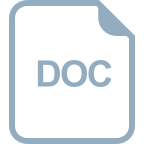
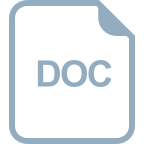




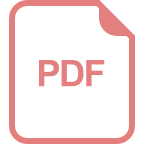
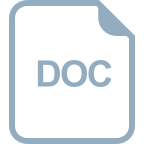
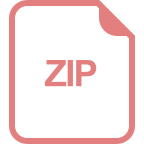
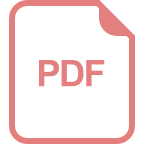
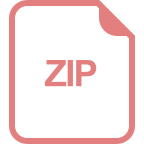
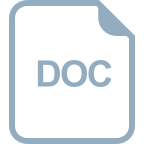
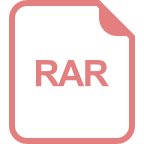
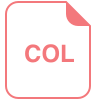
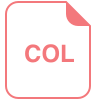


