Verilog 怎么实现函数传递数组
时间: 2024-03-10 12:48:54 浏览: 23
在 Verilog 中,你可以使用指针和 `for` 循环来实现函数传递数组。具体步骤如下:
1. 定义一个指针类型的参数,用于传递数组。
2. 在函数内部使用 `for` 循环遍历数组,并通过指针访问数组元素。
3. 在调用函数时,将数组的地址传递给指针参数。
下面是一个简单的例子:
```
module test(input [7:0] arr [0:3]);
// 定义一个指针类型参数
function automatic void test_func(input [7:0] *arr_ptr);
for (int i = 0; i < 4; i++) begin
$display("arr[%0d] = %0d", i, *arr_ptr++);
end
endfunction
initial begin
// 调用函数,并传递数组的地址
test_func(arr);
end
endmodule
```
在这个例子中,我们定义了一个名为 `test_func` 的函数,它接受一个指向 `arr` 数组的指针类型参数 `arr_ptr`。在函数内部,我们使用 `for` 循环遍历数组,并通过指针 `*arr_ptr` 访问数组元素。在调用函数时,我们传递了 `arr` 数组的地址作为参数。
需要注意的是,Verilog 中的数组访问是按地址顺序进行的,因此在函数内部使用指针访问数组元素时,要使用 `++` 运算符来移动指针的位置。
相关问题
verilog 可以用函数来传递数组吗
Verilog 是一种硬件描述语言,不支持函数传递数组。但是,你可以使用 SystemVerilog 扩展来支持函数传递数组。在 SystemVerilog 中,你可以使用数组引用参数和 $bits 来传递数组。例如:
```
module test(input logic [7:0] arr [0:3]);
function automatic void test_func(input logic [7:0] arr_ref [$]);
for(int i = 0; i < arr_ref.size(); i++) begin
$display("arr_ref[%0d] = %0d", i, $bits(arr_ref[i]));
end
endfunction
initial begin
test_func(arr);
end
endmodule
```
在这个例子中,我们定义了一个名为 `test_func` 的函数,它使用了一个数组引用参数 `arr_ref`,并且在 `initial` 块中调用了这个函数,并将 `arr` 作为参数传递。
verilog中实现数组
在Verilog中,可以使用以下语法来定义和实现数组:
```verilog
// 定义一个 8 位宽度,16 个元素的数组
reg [7:0] my_array [15:0];
// 初始化数组
initial begin
my_array[0] = 8'h01;
my_array[1] = 8'h02;
my_array[2] = 8'h03;
// ...
end
// 使用数组
always @ (posedge clk) begin
// 读取数组元素
data_out <= my_array[index];
// 写入数组元素
my_array[index] <= data_in;
end
```
上述代码定义了一个名为 `my_array` 的数组,它有 16 个元素,每个元素的宽度为 8 位。数组元素可以通过下标来访问,例如 `my_array[0]` 表示数组的第一个元素,`my_array[15]` 表示数组的最后一个元素。在初始化过程中,可以为数组的每个元素赋初值。在其他模块中,可以像使用普通变量一样使用数组变量,并且可以通过 `my_array[index]` 访问指定下标的元素。
相关推荐
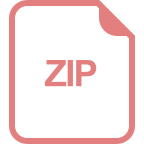












