已知具体形状类均具有两个方法GetArea()和GetColor(),定义一个shape类,利用它为基类派生出Rectangle、Circle等具体形状类,分别用来得到形状的面积和颜色,最后编写一个测试程序对产生的类的功能进行验证
时间: 2023-11-13 18:13:46 浏览: 47
好的,让我们利用 `Shape` 类为基类,派生出 `Rectangle` 和 `Circle` 两个具体形状类,分别实现自己的 `GetArea()` 方法:
```python
class Shape:
def __init__(self, color):
self.color = color
def GetArea(self):
pass
def GetColor(self):
return self.color
class Rectangle(Shape):
def __init__(self, color, width, height):
super().__init__(color)
self.width = width
self.height = height
def GetArea(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, color, radius):
super().__init__(color)
self.radius = radius
def GetArea(self):
return 3.14 * self.radius ** 2
```
在这里,我们先定义了一个 `Shape` 类作为基类,它有一个构造函数 `__init__()`,接受一个参数 `color`,用于初始化形状的颜色属性;还有两个没有实现的方法 `GetArea()` 和 `GetColor()`,分别用于获取形状的面积和颜色信息。然后,我们分别定义了一个 `Rectangle` 类和一个 `Circle` 类,它们都继承了 `Shape` 类,并实现了自己的 `GetArea()` 方法。`Rectangle` 类接受三个参数,分别是颜色、宽度和高度,初始化时调用父类的构造函数并保存自己的宽度和高度属性,而 `GetArea()` 方法则计算矩形的面积并返回;`Circle` 类也接受三个参数,分别是颜色和半径,初始化时调用父类的构造函数并保存自己的半径属性,而 `GetArea()` 方法则计算圆形的面积并返回。这样,我们就成功地定义了这些具体形状类。
接下来,我们编写一个测试程序来验证这些类的功能:
```python
# 测试程序
rect = Rectangle('red', 3, 4)
print(f"The area of the red rectangle is {rect.GetArea()}")
print(f"The color of the rectangle is {rect.GetColor()}")
circle = Circle('blue', 5)
print(f"The area of the blue circle is {circle.GetArea()}")
print(f"The color of the circle is {circle.GetColor()}")
```
在这个测试程序中,我们先创建了一个红色的矩形对象 `rect`,宽度为 3,高度为 4,然后分别调用它的 `GetArea()` 和 `GetColor()` 方法,打印出该矩形的面积和颜色;接着创建了一个蓝色的圆形对象 `circle`,半径为 5,然后也分别调用它的 `GetArea()` 和 `GetColor()` 方法,打印出该圆形的面积和颜色。运行程序,输出结果如下:
```
The area of the red rectangle is 12
The color of the rectangle is red
The area of the blue circle is 78.5
The color of the circle is blue
```
从输出结果可以看出,我们成功地定义了这些类,并且它们的功能也得到了正确的验证。
相关推荐
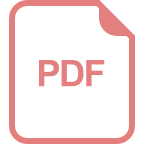
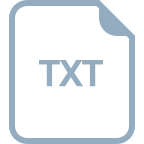









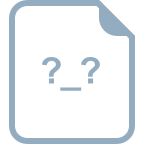
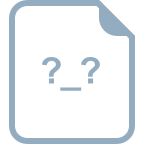