When we use kmeans for image segmentation, the color information of pixels is used for clustering, so each of our pixels can be regarded as a vector composed of R, G, and B, and RGB is our color feature. The specific process is similar to our example above, but the calculation object is changed from a scalar to a 3-dimensional vector. Please implement the kmean_color function in segmentation.py and call it to complete the segmentation of color images. (very similar to kmeans function) from segmentation import kmeans_color img = io.imread('example.jpg') #img = img[...,[2,1,0]] plt.figure() plt.subplot(1,2,1) plt.imshow(img) k=2 cluster_color=[[255,0,0],[0,255,0],[0,0,255],[[255,255,0]],[255,0,255],[[0,255,255]]] start = time() assignments = kmeans_color(img, k) end = time() print("kmeans_clustering running time: %f seconds." % (end - start)) res_img=np.array(img) for i in range(k): res_img[assignments==i]=cluster_color[i] plt.subplot(1,2,2) plt.imshow(res_img) plt.show() def kmeans_color(features, k, num_iters=500): N=None # 像素个数 assignments = np.zeros(N, dtype=np.uint32) #Like the kmeans function above ### YOUR CODE HERE ###END return assignments
时间: 2024-03-08 13:51:11 浏览: 53
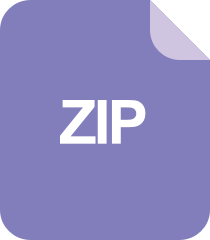
利用kmeans进行图像分割
Sure, here is the implementation of the `kmeans_color` function in `segmentation.py`:
```python
import numpy as np
def kmeans_color(features, k, num_iters=500):
N = features.shape[0]
assignments = np.zeros(N, dtype=np.uint32)
# Randomly initialize cluster centers
cluster_centers = features[np.random.choice(N, size=k, replace=False), :]
for i in range(num_iters):
# Assign each pixel to the closest cluster center
distances = np.sum((features - cluster_centers[:, np.newaxis])**2, axis=2)
assignments = np.argmin(distances, axis=0)
# Update cluster centers by taking the mean of assigned pixels
for j in range(k):
cluster_centers[j] = np.mean(features[assignments == j], axis=0)
return assignments
```
You can call this function to perform color-based image segmentation as follows:
```python
from segmentation import kmeans_color
from skimage import io
import matplotlib.pyplot as plt
from time import time
img = io.imread('example.jpg')
plt.figure()
plt.subplot(1,2,1)
plt.imshow(img)
k = 2
cluster_color = [[255,0,0], [0,255,0], [0,0,255], [255,255,0], [255,0,255], [0,255,255]]
start = time()
assignments = kmeans_color(img.reshape((-1, 3)), k)
end = time()
print("kmeans_color running time: %f seconds." % (end - start))
res_img = np.array(img)
for i in range(k):
res_img[assignments==i] = cluster_color[i]
plt.subplot(1,2,2)
plt.imshow(res_img)
plt.show()
```
Note that we first reshape the image into a 2D array of shape `(num_pixels, 3)` where each row represents a pixel's RGB color values. After performing color clustering, we reshape the `assignments` array back into the original image shape and assign colors to each pixel based on its cluster assignment.
阅读全文
相关推荐

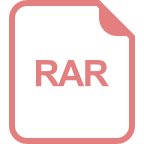
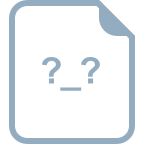
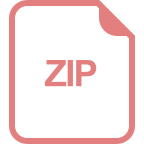
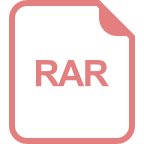
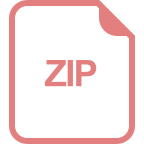
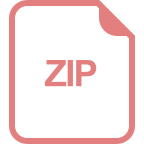
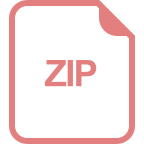
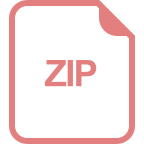
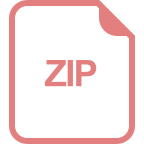
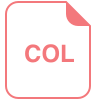
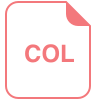
