解题排行榜中,按解题总数生成排行榜。假设每个学生信息仅包括学号、解题总数;要求先输入n个学生的信息;然后按“解题总数”降序排列,若“解题总数”相同则按“学号”升序排列。 输入格式: 首先输入一个正整数T,表示测试数据的组数,然后是T组测试数据。 每组测试数据先输入1个正整数n(1 ≤ n ≤ 100),表示学生总数。然后输入n行,每行包括1个不含空格的字符串s(不超过8位)和1个正整数d,分别表示一个学生的学号和解题总数。 输出格式: 对于每组测试数据,输出最终排名信息,每行一个学生的信息:排名、学号、解题总数。每行数据之间留一个空格。注意,解题总数相同的学生其排名也相同。用c语言编写
时间: 2023-06-19 20:10:56 浏览: 247
```
#include <stdio.h>
#include <string.h>
struct Student {
char id[10];
int score;
int rank; // 排名
};
// 比较函数,按照解题总数降序排列,若解题总数相同则按学号升序排列
int cmp(const void *a, const void *b) {
struct Student *s1 = (struct Student *)a;
struct Student *s2 = (struct Student *)b;
if (s1->score != s2->score) {
return s2->score - s1->score;
} else {
return strcmp(s1->id, s2->id);
}
}
int main() {
int t;
scanf("%d", &t);
while (t--) {
int n;
scanf("%d", &n);
struct Student stu[n];
for (int i = 0; i < n; i++) {
scanf("%s %d", stu[i].id, &stu[i].score);
}
// 排序
qsort(stu, n, sizeof(struct Student), cmp);
// 计算排名
int rank = 1;
for (int i = 0; i < n; i++) {
if (i == 0 || stu[i].score != stu[i-1].score) {
rank = i + 1;
}
stu[i].rank = rank;
}
// 输出结果
for (int i = 0; i < n; i++) {
printf("%d %s %d\n", stu[i].rank, stu[i].id, stu[i].score);
}
}
return 0;
}
```
阅读全文
相关推荐
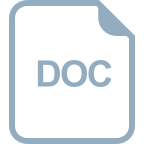
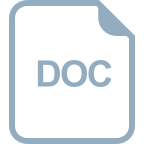
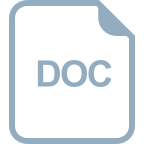















