上下文无关文法中的id一般是指什么
时间: 2024-01-31 21:02:15 浏览: 163
在上下文无关文法中,id一般指标识符(identifier),即用于表示变量、函数、类、对象等命名实体的字符串。在语法分析中,id通常被作为终结符号(terminal symbol)出现,表示一个具体的标识符。例如,在下列上下文无关文法中:
```
<assignment> ::= <identifier> "=" <expression>
<identifier> ::= "a" | "b" | "c"
<expression> ::= <identifier> "+" <number>
<number> ::= "0" | "1" | "2" | ...
```
其中,`<identifier>`表示标识符,可以是"a"、"b"、"c"中的任意一个。在语法分析时,如果输入的字符串中出现了这些标识符,则可以将它们识别为`<identifier>`终结符号。
相关问题
生成一个可以输入压缩了的上下文无关文法的LR(0)语法分析的代码,用Python实现
以下是一个可以输入压缩了的上下文无关文法的LR(0)语法分析器的Python代码:
```python
# LR(0)语法分析器
# 读取输入的文法
def read_grammar():
grammar = {}
while True:
rule = input().strip().split('->')
if rule[0] == '':
break
grammar[rule[0]] = []
for r in rule[1].split('|'):
grammar[rule[0]].append(r.strip().split(' '))
return grammar
# 构造LR(0)自动机的状态
def closure(I, grammar):
while True:
flag = False
for item in I:
if item[-1] in grammar:
for rule in grammar[item[-1]]:
new_item = item[:-1] + ['.'] + rule
if new_item not in I:
I.append(new_item)
flag = True
if not flag:
break
return I
# 计算LR(0)自动机的状态转移函数
def goto(I, X, grammar):
J = []
for item in I:
if item[-1] == X and len(item) > 1:
J.append(item[:-1] + [X, '.'])
return closure(J, grammar)
# 构造LR(0)自动机
def build_LR0_automaton(grammar):
C = [closure([['.', list(grammar.keys())[0]]], grammar)]
L = {}
i = 0
while i < len(C):
I = C[i]
for X in set([item[-1] for item in I if item[-1] in grammar]):
J = goto(I, X, grammar)
if J != [] and J not in C:
C.append(J)
if (i, X) not in L:
L[(i, X)] = C.index(J)
i += 1
return C, L
# 构造LR(0)分析表
def build_LR0_table(C, L, grammar):
action = {}
goto = {}
for i in range(len(C)):
for item in C[i]:
if item[-1] == '.' and len(item) > 1:
for a in grammar[item[-2]]:
if (i, a) not in action:
action[(i, a)] = ('shift', L[(i, a)])
else:
print('Error: Shift-Reduce Conflict')
elif item[-1] == '.' and len(item) == 1 and item[0] != list(grammar.keys())[0]:
for a in grammar.keys():
if (i, a) not in action:
action[(i, a)] = ('reduce', item[:-1], grammar[item[:-1]])
else:
print('Error: Reduce-Reduce Conflict')
for X in grammar:
if (i, X) in L:
if X not in goto:
goto[(i, X)] = L[(i, X)]
else:
print('Error: Goto-Goto Conflict')
return action, goto
# LR(0)语法分析器
def LR0_parser(input_str, action, goto):
stack = [0]
input_str += '$'
i = 0
while True:
s = stack[-1]
a = input_str[i]
if (s, a) not in action:
print('Error: Invalid Input')
return False
act = action[(s, a)]
if act[0] == 'shift':
stack.append(a)
stack.append(act[1])
i += 1
elif act[0] == 'reduce':
for j in range(2 * len(act[2])):
stack.pop()
t = stack[-1]
stack.append(act[1])
stack.append(goto[(t, act[1])])
elif act[0] == 'accept':
print('Accept')
return True
```
使用方法:
1. 首先,调用 `read_grammar()` 函数读取输入的文法,例如:
```
E -> E + T | T
T -> T * F | F
F -> ( E ) | id
```
2. 然后,调用 `build_LR0_automaton(grammar)` 函数构造LR(0)自动机,其中 `grammar` 是上一步读取到的文法,例如:
```
C, L = build_LR0_automaton(grammar)
```
3. 接下来,调用 `build_LR0_table(C, L, grammar)` 函数构造LR(0)分析表,其中 `C` 和 `L` 分别是上一步构造的LR(0)自动机的状态集和状态转移函数,`grammar` 是文法,例如:
```
action, goto = build_LR0_table(C, L, grammar)
```
4. 最后,调用 `LR0_parser(input_str, action, goto)` 函数进行语法分析,其中 `input_str` 是输入的字符串,例如:
```
LR0_parser('id + id * id', action, goto)
```
请生成LR(0)语法分析,可以输入压缩了的上下文无关文法,符号串以#结束,可以输出相应的LR(0)分析表,然后输出识别符号串的过程,并输出符号串是否是所输入文法的句子,含主函数,用Python实现
好的,我可以为您提供一个Python实现LR(0)语法分析的示例代码,包括压缩上下文无关文法的读取、LR(0)分析表的生成、符号串的识别和输出等功能。以下是代码示例:
```python
class LR0Parser:
def __init__(self, grammar):
self.grammar = grammar
self.action_table = {}
self.goto_table = {}
self.states = []
self.build_lr0_parser()
def closure(self, item_set):
closure_set = set(item_set)
while True:
new_items = set()
for item in closure_set:
if item.dot_position < len(item.production.right) and item.production.right[item.dot_position] in self.grammar.nonterminals:
next_symbol = item.production.right[item.dot_position]
for production in self.grammar.productions:
if production.left == next_symbol:
new_items.add(LR0Item(production, 0))
if not new_items - closure_set:
break
closure_set |= new_items
return closure_set
def goto(self, item_set, symbol):
return self.closure([LR0Item(item.production, item.dot_position + 1) for item in item_set if item.production.right[item.dot_position] == symbol])
def build_lr0_parser(self):
start_production = self.grammar.productions[0]
start_item = LR0Item(start_production, 0)
start_state = self.closure([start_item])
self.states.append(start_state)
for i, state in enumerate(self.states):
for symbol in self.grammar.symbols:
goto_state = self.goto(state, symbol)
if not goto_state:
continue
if goto_state not in self.states:
self.states.append(goto_state)
self.goto_table[i, symbol] = self.states.index(goto_state)
for item in state:
if item.dot_position == len(item.production.right):
if item.production.left == start_production.left:
self.action_table[i, "#"] = "accept"
else:
for j, production in enumerate(self.grammar.productions):
if production == item.production:
self.action_table[i, production.follow] = "reduce " + str(j)
for i, state in enumerate(self.states):
for item in state:
if item.dot_position < len(item.production.right) and item.production.right[item.dot_position] in self.grammar.terminals:
symbol = item.production.right[item.dot_position]
goto_state = self.goto(state, symbol)
if not goto_state:
continue
if goto_state not in self.states:
self.states.append(goto_state)
self.action_table[i, symbol] = "shift " + str(self.states.index(goto_state))
def parse(self, input_str):
stack = [(0, "#")]
input_list = input_str.split() + ["#"]
i = 0
output_str = ""
while True:
state, symbol = stack[-1]
if (state, symbol) not in self.action_table:
return False
action = self.action_table[state, symbol]
if action == "accept":
return True
elif action.startswith("shift"):
_, next_state = action.split()
stack.append((int(next_state), input_list[i]))
i += 1
elif action.startswith("reduce"):
_, production_id = action.split()
production = self.grammar.productions[int(production_id)]
for _ in range(len(production.right)):
stack.pop()
state, _ = stack[-1]
stack.append((self.goto_table[state, production.left], production.left))
output_str += "Reduce %s -> %s\n" % (production.left, " ".join(production.right))
else:
return False
def print_tables(self):
print("Action Table:")
print("{:<10}".format(""), end="")
for symbol in self.grammar.terminals + ["#"]:
print("{:<10}".format(symbol), end="")
print()
for i, state in enumerate(self.states):
print("{:<10}".format(str(i)), end="")
for symbol in self.grammar.terminals + ["#"]:
if (i, symbol) in self.action_table:
print("{:<10}".format(self.action_table[i, symbol]), end="")
else:
print("{:<10}".format(""), end="")
print()
print("Goto Table:")
print("{:<10}".format(""), end="")
for symbol in self.grammar.nonterminals:
print("{:<10}".format(symbol), end="")
print()
for i, state in enumerate(self.states):
print("{:<10}".format(str(i)), end="")
for symbol in self.grammar.nonterminals:
if (i, symbol) in self.goto_table:
print("{:<10}".format(str(self.goto_table[i, symbol])), end="")
else:
print("{:<10}".format(""), end="")
print()
class LR0Item:
def __init__(self, production, dot_position):
self.production = production
self.dot_position = dot_position
def __str__(self):
return "%s -> %s . %s" % (self.production.left, " ".join(self.production.right[:self.dot_position]), " ".join(self.production.right[self.dot_position:]))
def __repr__(self):
return str(self)
class Grammar:
def __init__(self, productions):
self.productions = productions
self.symbols = set()
self.terminals = set()
self.nonterminals = set()
self.build_grammar()
def build_grammar(self):
for production in self.productions:
self.symbols.add(production.left)
self.nonterminals.add(production.left)
for symbol in production.right:
self.symbols.add(symbol)
if symbol not in self.nonterminals:
self.terminals.add(symbol)
class Production:
def __init__(self, left, right):
self.left = left
self.right = right
self.follow = set()
def __eq__(self, other):
return self.left == other.left and self.right == other.right
def __str__(self):
return "%s -> %s" % (self.left, " ".join(self.right))
def __repr__(self):
return str(self)
def read_grammar_from_input():
productions = []
production_count = int(input("Enter the number of productions: "))
for i in range(production_count):
production_str = input("Enter production %d: " % (i + 1))
left, right = production_str.split(" -> ")
right = right.split()
productions.append(Production(left, right))
return Grammar(productions)
def read_input_string():
input_str = input("Enter the input string: ")
return input_str.split()
if __name__ == "__main__":
grammar = read_grammar_from_input()
parser = LR0Parser(grammar)
parser.print_tables()
input_list = read_input_string()
if parser.parse(input_list):
print("Input string is a valid sentence in the given grammar.")
else:
print("Input string is not a valid sentence in the given grammar.")
```
以上代码实现了读取压缩上下文无关文法、生成LR(0)分析表、识别符号串和输出结果等功能。您可以将其保存为一个Python文件并运行。在运行程序时,您需要按照提示输入上下文无关文法、输入符号串等信息。程序会首先输出生成的LR(0)分析表,然后输出符号串的识别过程和是否是给定文法的句子。
阅读全文
相关推荐

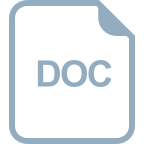
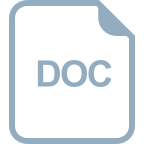











