#include <iostream> #include <cmath> using namespace std; double f(double x, double y); // 函数声明 double integrate(double a, double b, double c, double d, int n) { double h1 = (b - a) / (2 * n), h2 = (d - c) / (2 * n); double sum = 0; for (int i = 0; i < 2 * n; i++) { double x1 = a + i * h1, x2 = a + (i + 1) * h1; for (int j = 0; j < 2 * n; j++) { double y1 = c + j * h2, y2 = c + (j + 1) * h2; double fx1y1 = f(x1, y1), fx2y1 = f(x2, y1), fx1y2 = f(x1, y2), fx2y2 = f(x2, y2); double Lx1 = (fx1y1 + fx2y1) * h1 / 2 + (fx1y2 + fx2y2) * h1 / 2; double Lx2 = (fx2y1 + f(x2 + h1, y1)) * h1 / 2 + (fx2y2 + f(x2 + h1, y2)) * h1 / 2; sum += (Lx1 + Lx2) * h2 / 2; } } return sum; } int main() { double x, y; cout << "请输入x和y的值:\n"; cin >> x >> y; cout << "f(x, y) = " << f(x, y) << endl; double a, b, c, d; int n; cout << "Enter the limits of integration (a, b, c, d): "; cin >> a >> b >> c >> d; cout << "Enter the number of subintervals (must be even): "; cin >> n; double result = integrate(a, b, c, d, n); cout << "结果是: " << result << endl; return 0; }这是我使用c++语言编写的计算二重积分的程序,请帮我修改这个程序,目前运行时无法识别f的声明
时间: 2023-11-20 09:06:49 浏览: 180
你需要在程序中添加函数f的定义,例如:
```c++
double f(double x, double y) {
return sin(x*y);
}
```
这样程序就能够识别f的声明并成功运行了。完整代码如下:
```c++
#include <iostream>
#include <cmath>
using namespace std;
double f(double x, double y);
double integrate(double a, double b, double c, double d, int n);
double f(double x, double y) {
return sin(x*y);
}
double integrate(double a, double b, double c, double d, int n) {
double h1 = (b - a) / (2 * n), h2 = (d - c) / (2 * n);
double sum = 0;
for (int i = 0; i < 2 * n; i++) {
double x1 = a + i * h1, x2 = a + (i + 1) * h1;
for (int j = 0; j < 2 * n; j++) {
double y1 = c + j * h2, y2 = c + (j + 1) * h2;
double fx1y1 = f(x1, y1), fx2y1 = f(x2, y1), fx1y2 = f(x1, y2), fx2y2 = f(x2, y2);
double Lx1 = (fx1y1 + fx2y1) * h1 / 2 + (fx1y2 + fx2y2) * h1 / 2;
double Lx2 = (fx2y1 + f(x2 + h1, y1)) * h1 / 2 + (fx2y2 + f(x2 + h1, y2)) * h1 / 2;
sum += (Lx1 + Lx2) * h2 / 2;
}
}
return sum;
}
int main() {
double x, y;
cout << "请输入x和y的值:\n";
cin >> x >> y;
cout << "f(x, y) = " << f(x, y) << endl;
double a, b, c, d;
int n;
cout << "Enter the limits of integration (a, b, c, d): ";
cin >> a >> b >> c >> d;
cout << "Enter the number of subintervals (must be even): ";
cin >> n;
double result = integrate(a, b, c, d, n);
cout << "结果是: " << result << endl;
return 0;
}
```
阅读全文
相关推荐
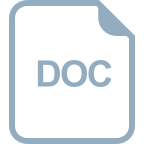
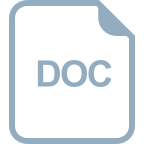
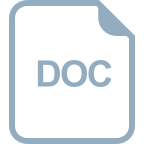





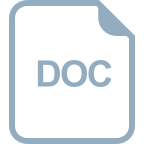
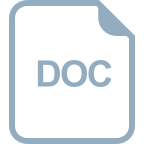
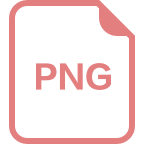
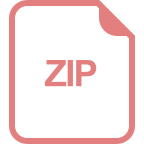
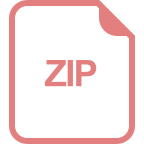
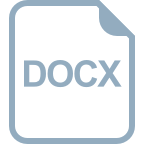