优化代码:def save_file(data, out_path): if not os.path.exists(out_path): os.mkdir(out_path) with open(out_path, 'w') as f: json.dump(data, f)
时间: 2024-03-17 11:44:34 浏览: 110
可以尝试使用os.makedirs()代替os.mkdir(),因为前者能够递归创建路径中的所有文件夹。
同时,建议添加异常处理,以确保在发生错误时代码不会崩溃。例如,可以使用try-except语句来捕获文件打开或写入错误,并进行相应处理。最好将错误信息记录到日志文件中,以便后续查看和调试。
修改后的代码如下:
```python
import os
import json
def save_file(data, out_path):
try:
os.makedirs(out_path, exist_ok=True)
with open(out_path, 'w', encoding='utf-8') as f:
json.dump(data, f, ensure_ascii=False)
except Exception as e:
# 记录错误信息到日志文件中
with open('error.log', 'a', encoding='utf-8') as f:
f.write(f'Error in save_file: {str(e)}\n')
```
其中,`exist_ok=True`表示如果目录已经存在则不会报错,`ensure_ascii=False`表示以utf-8编码写入json文件,避免中文出现乱码。同时,异常处理语句可以根据具体情况进行修改和优化。
相关问题
import requests import urllib.request import os def quest_find(quest_url, awme_id): params = {"id": awme_id} respon = requests.get(quest_url, params=params).json() return respon["data"], respon["code"] def re_down(url,filename): try: urllib.request.urlretrieve(url,filename) except urllib.error.ContentTooShortError: print ('Network conditions is not good. Reloading...') re_down(url,filename) # 获取视频URL,并下载 if __name__ == '__main__': quest_url = "http://discover-rpc.cmm-crawler-intranet.k8s.limayao.com/play_url" save_path = "/home/algodev/sujunbin/whisper/test_model/video%s" %time if not os.path.exists(save_path): os.mkdir(save_path) awme_ids = ['7119114587735100687'] with open('id_time.txt','r') as file: for line in file.readlines(): line = line.split() id = line[0] time1 = int(line[1]) if time1<10000: time ='<10s' elif 10000<=time1<20000: time='10-20s' elif 20000<=time1<30000: time='20-30s' elif 30000<=time1<40000: time='30-40s' elif 40000<=time1<50000: time='40-50s' elif 50000<=time1<60000: time='50-60s' elif 60000<=time1<90000: time='60-90s' elif 90000<=time1<120000: time='90-120s' elif 120000<=time1<180000: time='120-180s' elif time1>=180000: time='>180s' save_path = "/home/algodev/sujunbin/whisper/test_model/video%s" %time if not os.path.exists(save_path): os.mkdir(save_path) data_json, code = quest_find(quest_url, id) play_url = data_json['play_url'] video_name = id + '.mp4' save_video_path = os.path.join(save_path, video_name) re_down(data_json['play_url'], save_video_path) print(save_video_path) for i in range(len(awme_ids)): data_json, code = quest_find(quest_url, awme_ids[i]) play_url = data_json['play_url'] video_name = awme_ids[i] + '.mp4' save_video_path = os.path.join(save_path, video_name) urllib.request.urlretrieve(data_json['play_url'], save_video_path) print(save_video_path) print("done!")这段代码有什么问题
这段代码可能存在以下问题:
1. 缺少必要的模块导入语句,需要添加 `import time`。
2. 代码中的变量名 `awme_ids` 可能有误,应该改为 `aweme_ids`。
3. 在 `re_down()` 函数中,若下载失败会无限递归调用自身,可能导致内存溢出。建议添加一个计数器,若连续下载失败次数超过一定阈值,则放弃下载。
4. 在循环过程中,多次声明 `save_path` 变量,覆盖了之前的赋值,应该在循环外部声明。
5. 可能存在无法下载视频的情况,需要添加异常处理机制,例如 `urllib.error.HTTPError`、`urllib.error.URLError`、`socket.timeout` 等异常。
6. 代码中的请求 URL 是内网地址,无法在公网环境下使用。
7. 可能存在多个视频对应同一个保存路径的情况,应该在视频名中添加一个唯一标识,例如时间戳或视频 ID。
建议在代码中添加适当的注释和异常处理,以提高代码的可读性和健壮性。
import tkinter as tk import pandas as pd import matplotlib.pyplot as plt from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg import os class ExcelPlotter(tk.Frame): def init(self, master=None): super().init(master) self.master = master self.master.title("图方便") self.file_label = tk.Label(master=self, text="Excel File Path:") self.file_label.grid(row=0, column=0, sticky="w") self.file_entry = tk.Entry(master=self) self.file_entry.grid(row=0, column=1, columnspan=2, sticky="we") self.file_button = tk.Button(master=self, text="Open", command=self.open_file) self.file_button.grid(row=0, column=3, sticky="e") self.plot_button = tk.Button(master=self, text="Plot", command=self.plot_data) self.plot_button.grid(row=1, column=2, sticky="we") self.name_label = tk.Label(master=self, text="Out Image Name:") self.name_label.grid(row=2, column=0, sticky="w") self.name_entry = tk.Entry(master=self) self.name_entry.grid(row=2, column=1, columnspan=2, sticky="we") self.save_button = tk.Button(master=self, text="Save", command=self.save_image) self.save_button.grid(row=2, column=3, sticky="e") self.figure = plt.figure(figsize=(5, 4), dpi=150) self.canvas = FigureCanvasTkAgg(self.figure, master=self) self.canvas.get_tk_widget().grid(row=4, column=0, columnspan=4, sticky="we") self.pack() def open_file(self): file_path = tk.filedialog.askopenfilename(filetypes=[("Excel Files", "*.xls")]) self.file_entry.delete(0, tk.END) self.file_entry.insert(tk.END, file_path) def plot_data(self): file_path = self.file_entry.get() if os.path.exists(file_path): data = pd.read_excel(file_path) plt.plot(data['波长(nm)'], data['吸光度'], 'k') plt.xlim(300, 1000) plt.xlabel('Wavelength(nm)', fontsize=16) plt.ylabel('Abs.', fontsize=16) plt.gcf().subplots_adjust(left=0.13, top=0.91, bottom=0.16) plt.savefig('Last Fig', dpi=1000) plt.show() def save_image(self): if self.figure: file_path = tk.filedialog.asksaveasfilename(defaultextension=".png") if file_path: self.figure.savefig(file_path) root = tk.Tk() app = ExcelPlotter(master=root) app.mainloop()帮我增加一个删除当前图像的功能
好的,您可以在 `ExcelPlotter` 类中添加一个名为 `delete_image` 的方法,实现删除当前图像的功能。可以在该方法中调用 `self.figure.clf()` 清空当前图像,然后在界面上更新显示。下面是修改后的代码:
```python
import tkinter as tk
import pandas as pd
import matplotlib.pyplot as plt
from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg
import os
class ExcelPlotter(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.master.title("图方便")
self.file_label = tk.Label(master=self, text="Excel File Path:")
self.file_label.grid(row=0, column=0, sticky="w")
self.file_entry = tk.Entry(master=self)
self.file_entry.grid(row=0, column=1, columnspan=2, sticky="we")
self.file_button = tk.Button(master=self, text="Open", command=self.open_file)
self.file_button.grid(row=0, column=3, sticky="e")
self.plot_button = tk.Button(master=self, text="Plot", command=self.plot_data)
self.plot_button.grid(row=1, column=2, sticky="we")
self.delete_button = tk.Button(master=self, text="Delete", command=self.delete_image)
self.delete_button.grid(row=1, column=3, sticky="e")
self.name_label = tk.Label(master=self, text="Out Image Name:")
self.name_label.grid(row=2, column=0, sticky="w")
self.name_entry = tk.Entry(master=self)
self.name_entry.grid(row=2, column=1, columnspan=2, sticky="we")
self.save_button = tk.Button(master=self, text="Save", command=self.save_image)
self.save_button.grid(row=2, column=3, sticky="e")
self.figure = plt.figure(figsize=(5, 4), dpi=150)
self.canvas = FigureCanvasTkAgg(self.figure, master=self)
self.canvas.get_tk_widget().grid(row=4, column=0, columnspan=4, sticky="we")
self.pack()
def open_file(self):
file_path = tk.filedialog.askopenfilename(filetypes=[("Excel Files", "*.xls")])
self.file_entry.delete(0, tk.END)
self.file_entry.insert(tk.END, file_path)
def plot_data(self):
file_path = self.file_entry.get()
if os.path.exists(file_path):
data = pd.read_excel(file_path)
plt.plot(data['波长(nm)'], data['吸光度'], 'k')
plt.xlim(300, 1000)
plt.xlabel('Wavelength(nm)', fontsize=16)
plt.ylabel('Abs.', fontsize=16)
plt.gcf().subplots_adjust(left=0.13, top=0.91, bottom=0.16)
plt.show()
def delete_image(self):
if self.figure:
self.figure.clf()
self.canvas.draw()
def save_image(self):
if self.figure:
file_path = tk.filedialog.asksaveasfilename(defaultextension=".png")
if file_path:
self.figure.savefig(file_path)
root = tk.Tk()
app = ExcelPlotter(master=root)
app.mainloop()
```
在界面上添加了一个名为 `Delete` 的按钮,点击该按钮会调用 `delete_image` 方法。该方法会清空当前图像并在界面上更新显示。
阅读全文
相关推荐
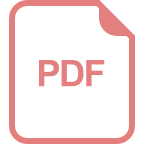
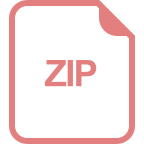









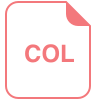
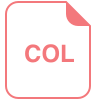
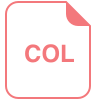
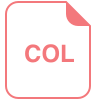
