如何用python求最短路径
时间: 2024-03-23 21:37:28 浏览: 14
可以使用Python中的networkx库来求解图的最短路径。以下是一个示例代码:
```python
import networkx as nx
# 创建一个有向图
G = nx.DiGraph()
# 添加节点
G.add_node('A')
G.add_node('B')
G.add_node('C')
G.add_node('D')
G.add_node('E')
# 添加边及其权重
G.add_edge('A', 'B', weight=3)
G.add_edge('A', 'C', weight=2)
G.add_edge('B', 'D', weight=4)
G.add_edge('C', 'D', weight=1)
G.add_edge('C', 'E', weight=5)
G.add_edge('D', 'E', weight=1)
# 计算A到E的最短路径
shortest_path = nx.shortest_path(G, 'A', 'E', weight='weight')
# 输出结果
print(shortest_path)
```
输出结果为:
```
['A', 'C', 'D', 'E']
```
其中,列表中的每个元素代表路径上的一个节点。这个示例中的图是一个有向图,如果你需要计算无向图的最短路径,只需要将`nx.DiGraph()`改为`nx.Graph()`即可。
相关问题
python求最短路径
Python中求最短路径的方法有很多,其中一种比较常用的方法是使用Dijkstra算法。Dijkstra算法是一种贪心算法,用于解决带有非负权值边的最短路径问题。以下是一个使用Dijkstra算法的示例代码:
```python
import heapq
def dijkstra(graph, start):
distances = {node: float('inf') for node in graph}
distances[start] = 0
queue = [(0, start)]
visited = set()
while queue:
curr_distance, curr_node = heapq.heappop(queue)
if curr_node in visited:
continue
visited.add(curr_node)
for neighbor, weight in graph[curr_node].items():
distance = curr_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances
```
在这个示例代码中,使用了一个字典来表示图,其中字典的键表示节点,字典的值也是一个字典,用来表示该节点到它的邻居节点的边的权值。函数的参数`start`表示起始节点。
该函数返回一个字典,表示从起始节点到每个节点的最短距离。
dijkstra算法python求最短路径
以下是使用Python实现Dijkstra算法求最短路径的示例代码:
```python
import heapq
def dijkstra(graph, start, end):
# 初始化距离字典和前驱节点字典
distances = {vertex: float('inf') for vertex in graph}
distances[start] = 0
previous_vertices = {vertex: None for vertex in graph}
# 将起始节点加入堆中
vertices = [(0, start)]
heapq.heapify(vertices)
while vertices:
# 取出堆中距离最小的节点
current_distance, current_vertex = heapq.heappop(vertices)
# 如果当前节点已经处理过,跳过
if current_distance > distances[current_vertex]:
continue
# 遍历当前节点的邻居节点
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
# 如果发现更短的路径,则更新距离和前驱节点
if distance < distances[neighbor]:
distances[neighbor] = distance
previous_vertices[neighbor] = current_vertex
# 将邻居节点加入堆中
heapq.heappush(vertices, (distance, neighbor))
# 构造最短路径
path = []
vertex = end
while vertex is not None:
path.append(vertex)
vertex = previous_vertices[vertex]
path.reverse()
return path, distances[end]
# 示例
graph = {
'A': {'B': 2, 'C': 1},
'B': {'A': 2, 'D': 3, 'E': 2},
'C': {'A': 1, 'F': 4},
'D': {'B': 3},
'E': {'B': 2, 'F': 3},
'F': {'C': 4, 'E': 3}
}
start = 'A'
end = 'F'
path, distance = dijkstra(graph, start, end)
print('最短路径:', path)
print('最短距离:', distance)
```
相关推荐
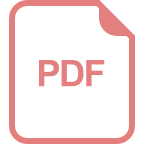
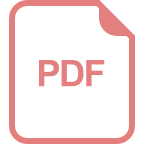
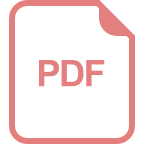












