三线性插值c++代码实现
时间: 2024-03-15 21:22:44 浏览: 13
下面是一个简单的三线性插值的C++代码实现,假设需要在一个三维网格的数据中进行插值:
```C++
#include <iostream>
#include <vector>
using namespace std;
// 定义一个三维向量类
class Vector3D {
public:
float x, y, z;
Vector3D(float _x, float _y, float _z) : x(_x), y(_y), z(_z) {}
};
// 定义一个三维网格类
class Grid {
public:
int nx, ny, nz; // 网格的尺寸
vector<float> data; // 存储三维数据的向量
Grid(int _nx, int _ny, int _nz) : nx(_nx), ny(_ny), nz(_nz) {
data.resize(nx * ny * nz); // 初始化数据向量
}
// 获取某个位置的数据
float get(int ix, int iy, int iz) const {
return data[iz * nx * ny + iy * nx + ix];
}
// 设置某个位置的数据
void set(int ix, int iy, int iz, float value) {
data[iz * nx * ny + iy * nx + ix] = value;
}
// 计算插值
float interpolate(float x, float y, float z) const {
int i0 = (int)x;
int i1 = i0 + 1;
int j0 = (int)y;
int j1 = j0 + 1;
int k0 = (int)z;
int k1 = k0 + 1;
float dx = x - i0;
float dy = y - j0;
float dz = z - k0;
float c000 = get(i0, j0, k0);
float c001 = get(i0, j0, k1);
float c010 = get(i0, j1, k0);
float c011 = get(i0, j1, k1);
float c100 = get(i1, j0, k0);
float c101 = get(i1, j0, k1);
float c110 = get(i1, j1, k0);
float c111 = get(i1, j1, k1);
float c00 = c000 * (1 - dx) + c100 * dx;
float c01 = c001 * (1 - dx) + c101 * dx;
float c10 = c010 * (1 - dx) + c110 * dx;
float c11 = c011 * (1 - dx) + c111 * dx;
float c0 = c00 * (1 - dy) + c10 * dy;
float c1 = c01 * (1 - dy) + c11 * dy;
return c0 * (1 - dz) + c1 * dz;
}
};
int main() {
Grid grid(3, 3, 3); // 创建一个3x3x3的网格
// 初始化网格中的数据
grid.set(0, 0, 0, 0);
grid.set(0, 0, 1, 1);
grid.set(0, 1, 0, 2);
grid.set(0, 1, 1, 3);
grid.set(1, 0, 0, 4);
grid.set(1, 0, 1, 5);
grid.set(1, 1, 0, 6);
grid.set(1, 1, 1, 7);
// 进行插值
float value = grid.interpolate(1.5, 1.5, 1.5);
cout << "Interpolated value: " << value << endl;
return 0;
}
```
上述代码中,我们定义了一个三维向量类和一个三维网格类,其中网格类包含了一个三维向量的数据。在插值函数中,我们首先根据输入的坐标值计算出八个数据点的位置,然后根据三线性插值公式计算出插值结果。最后我们可以通过调用插值函数来获取任意三维坐标位置的插值结果。
相关推荐
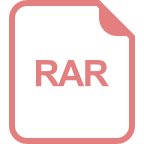














