先创建一个Point类,然后定义Triangle类。在Triangle类中定义三个Point的对象用来表示一个三角形的三个点,用构造方法对这三个点进行初始化,然后定义两个方法求三角形的周长、面积。在main()中创建一个三
时间: 2023-08-31 16:28:47 浏览: 70
### 回答1:
角形对象并调用方法计算周长和面积。
代码如下:
```java
class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
}
class Triangle {
private Point pointA;
private Point pointB;
private Point pointC;
public Triangle(Point pointA, Point pointB, Point pointC) {
this.pointA = pointA;
this.pointB = pointB;
this.pointC = pointC;
}
public double getLength(Point p1, Point p2) {
double length = Math.sqrt(Math.pow(p1.getX()-p2.getX(), 2) + Math.pow(p1.getY()-p2.getY(), 2));
return length;
}
public double getPerimeter() {
double lengthAB = getLength(pointA, pointB);
double lengthBC = getLength(pointB, pointC);
double lengthCA = getLength(pointC, pointA);
double perimeter = lengthAB + lengthBC + lengthCA;
return perimeter;
}
public double getArea() {
double lengthAB = getLength(pointA, pointB);
double lengthBC = getLength(pointB, pointC);
double lengthCA = getLength(pointC, pointA);
double s = (lengthAB + lengthBC + lengthCA) / 2;
double area = Math.sqrt(s * (s-lengthAB) * (s-lengthBC) * (s-lengthCA));
return area;
}
}
public class Main {
public static void main(String[] args) {
Point pointA = new Point(0, 0);
Point pointB = new Point(4, 0);
Point pointC = new Point(0, 3);
Triangle triangle = new Triangle(pointA, pointB, pointC);
System.out.println("周长为:" + triangle.getPerimeter());
System.out.println("面积为:" + triangle.getArea());
}
}
```
输出结果:
```
周长为:12.0
面积为:6.0
```
### 回答2:
首先,我们需要创建一个Point类来表示点的坐标。Point类可以包含两个私有属性x和y,分别表示点的横坐标和纵坐标。我们可以定义一个构造方法来初始化这两个属性。
接下来,我们可以定义Triangle类来表示三角形。Triangle类可以包含三个Point对象,分别表示三角形的三个顶点。我们可以定义一个构造方法来初始化这三个顶点。
在Triangle类中,我们可以定义两个方法来求三角形的周长和面积。求周长的方法可以计算三个顶点之间的距离之和,而求面积的方法可以利用三个顶点的坐标来进行计算。
最后,在main()方法中,我们可以创建一个Triangle对象来表示一个三角形。通过调用Triangle类中的方法,我们可以求出这个三角形的周长和面积,并将结果输出。
下面是一个示例的代码实现:
```java
class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
}
class Triangle {
private Point point1;
private Point point2;
private Point point3;
public Triangle(Point point1, Point point2, Point point3) {
this.point1 = point1;
this.point2 = point2;
this.point3 = point3;
}
public double getPerimeter() {
double distance1 = Math.sqrt(Math.pow(point2.getX()-point1.getX(), 2) + Math.pow(point2.getY()-point1.getY(), 2));
double distance2 = Math.sqrt(Math.pow(point3.getX()-point2.getX(), 2) + Math.pow(point3.getY()-point2.getY(), 2));
double distance3 = Math.sqrt(Math.pow(point1.getX()-point3.getX(), 2) + Math.pow(point1.getY()-point3.getY(), 2));
return distance1 + distance2 + distance3;
}
public double getArea() {
double area = Math.abs((point1.getX()*(point2.getY()-point3.getY()) + point2.getX()*(point3.getY()-point1.getY()) + point3.getX()*(point1.getY()-point2.getY())) / 2);
return area;
}
}
public class Main {
public static void main(String[] args) {
Point point1 = new Point(0, 0);
Point point2 = new Point(0, 4);
Point point3 = new Point(3, 0);
Triangle triangle = new Triangle(point1, point2, point3);
double perimeter = triangle.getPerimeter();
double area = triangle.getArea();
System.out.println("三角形的周长为:" + perimeter);
System.out.println("三角形的面积为:" + area);
}
}
```
以上代码中,我们创建了一个Point类来表示一个点的坐标,然后定义了一个Triangle类来表示一个三角形。在main()方法中,我们创建了一个三角形对象,并通过调用Triangle类中的方法求出了三角形的周长和面积,并将结果输出。
### 回答3:
首先我们需要创建一个Point类,用来表示一个点的坐标。
```java
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
}
```
接下来定义一个Triangle类,其中包含三个Point对象,并通过构造方法对这三个点进行初始化。
```java
public class Triangle {
private Point point1;
private Point point2;
private Point point3;
public Triangle(Point point1, Point point2, Point point3) {
this.point1 = point1;
this.point2 = point2;
this.point3 = point3;
}
public double getPerimeter() {
double side1 = calculateDistance(point1, point2);
double side2 = calculateDistance(point2, point3);
double side3 = calculateDistance(point3, point1);
return side1 + side2 + side3;
}
public double getArea() {
double side1 = calculateDistance(point1, point2);
double side2 = calculateDistance(point2, point3);
double side3 = calculateDistance(point3, point1);
double s = (side1 + side2 + side3) / 2; // 半周长
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3)); // 海伦公式求面积
}
// 计算两点间的距离
private double calculateDistance(Point p1, Point p2) {
double x1 = p1.getX();
double y1 = p1.getY();
double x2 = p2.getX();
double y2 = p2.getY();
return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2));
}
}
```
在main()函数中,我们可以创建一个Triangle对象,并调用其中的方法得到三角形的周长和面积。
```java
public class Main {
public static void main(String[] args) {
Point point1 = new Point(0, 0);
Point point2 = new Point(0, 1);
Point point3 = new Point(1, 0);
Triangle triangle = new Triangle(point1, point2, point3);
double perimeter = triangle.getPerimeter();
double area = triangle.getArea();
System.out.println("三角形的周长:" + perimeter);
System.out.println("三角形的面积:" + area);
}
}
```
这样就可以得到一个点坐标分别为(0,0)、(0,1)、(1,0)的三角形的周长和面积。
相关推荐
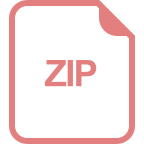
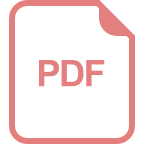














