能实现读取一篇英文文章基于线性表实现单词的词频统计与查找的C语言代码
时间: 2023-03-24 07:00:21 浏览: 115
好的,以下是基于线性表实现单词频率统计和查找的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 50 // 单词的最大长度
#define MAX_WORD_NUM 1000 // 最多可以统计的单词数
// 定义一个结构体来表示一个单词及其出现次数
struct word_freq {
char word[MAX_WORD_LEN];
int freq;
};
// 判断一个字符是否为单词分隔符(这里只考虑了空格和换行符)
int is_word_separator(char c) {
return c == ' ' || c == '\n';
}
// 将一个字符串转化为小写字母
void to_lower_case(char *str) {
int i;
for (i = 0; str[i]; i++) {
str[i] = tolower(str[i]);
}
}
// 统计一个字符串中的单词频率
void count_word_freq(char *str, struct word_freq *freq_array, int *num_words) {
char *word_start, *word_end;
int i, j, len, found;
word_start = str;
while (*word_start) {
// 找到下一个单词的起始位置
while (*word_start && is_word_separator(*word_start)) {
word_start++;
}
// 如果到了字符串末尾,则退出循环
if (!*word_start) {
break;
}
// 找到下一个单词的结束位置
word_end = word_start + 1;
while (*word_end && !is_word_separator(*word_end)) {
word_end++;
}
// 将单词复制到一个新的字符串中,并将其转化为小写字母
len = word_end - word_start;
char *word = (char *)malloc((len + 1) * sizeof(char));
strncpy(word, word_start, len);
word[len] = '\0';
to_lower_case(word);
// 在已有的单词中查找该单词
found = 0;
for (i = 0; i < *num_words; i++) {
if (strcmp(freq_array[i].word, word) == 0) {
freq_array[i].freq++;
found = 1;
break;
}
}
// 如果没有找到,则将该单词添加到数组中
if (!found) {
if (*num_words >= MAX_WORD_NUM) {
printf("Error: too many words!\n");
exit(1);
}
strncpy(freq_array[*num_words].word, word, MAX_WORD_LEN);
freq_array[*num_words].freq = 1;
(*num_words)++;
}
// 释放空间
free(word);
// 将指针指向下一个单词
word_start = word_end + 1;
}
}
// 按照单词出现次数从高到低排序
void sort_word_freq(struct word_freq *freq_array, int num_words) {
int i, j;
struct word_freq tmp;
for (i = 0; i < num_words - 1; i++) {
for (j = i + 1; j < num_words; j++)
阅读全文
相关推荐
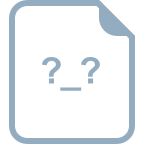

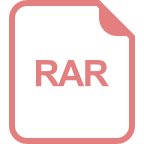
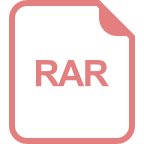
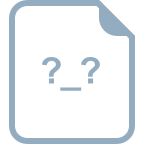
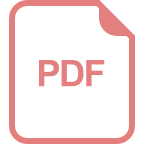
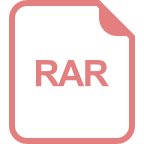
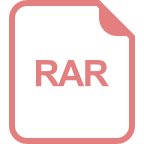
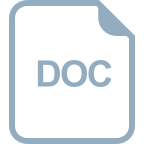
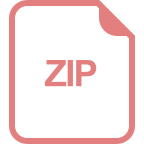
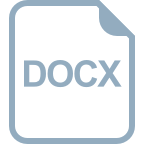
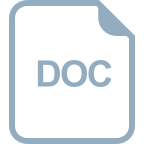
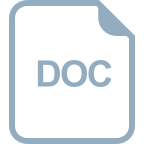
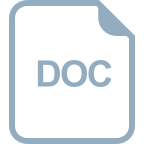
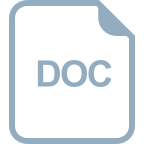