请在Linux利用c语言编写程序,使用共享内存在进程A和进程B之间发送数据,进程A向进程B发送hello,进程B收到进程A的数据hello后发送world给进程A...
时间: 2024-05-14 11:18:50 浏览: 81
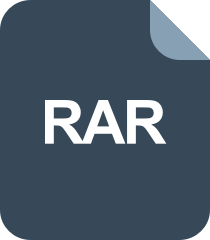
一个C语言的helloworld程序
以下是一个简单的C语言程序,使用共享内存在进程A和进程B之间发送数据。其中,进程A向共享内存中写入字符串“hello”,然后等待进程B写入字符串“world”。进程B从共享内存中读取字符串“hello”,然后向共享内存中写入字符串“world”:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <unistd.h>
#define SHM_SIZE 1024
int main() {
int shmid;
char *shm_ptr;
char *data;
// 创建共享内存段
if ((shmid = shmget(IPC_PRIVATE, SHM_SIZE, IPC_CREAT|0666)) < 0) {
perror("shmget");
exit(1);
}
// 将共享内存段附加到进程空间
if ((shm_ptr = shmat(shmid, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
// 进程A向共享内存中写入数据
data = "hello";
strncpy(shm_ptr, data, strlen(data));
// 进程B从共享内存中读取数据
while (strncmp(shm_ptr, "world", 5) != 0) {
sleep(1);
}
// 进程B向共享内存中写入数据
data = "world";
strncpy(shm_ptr, data, strlen(data));
// 将共享内存段从进程空间中分离
if (shmdt(shm_ptr) == -1) {
perror("shmdt");
exit(1);
}
// 删除共享内存段
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(1);
}
return 0;
}
```
编译并运行该程序,使用两个终端窗口分别运行进程A和进程B:
```bash
$ gcc -o share_memory share_memory.c
$ ./share_memory
```
进程A将向共享内存中写入字符串“hello”,然后等待进程B写入字符串“world”。进程B从共享内存中读取字符串“hello”,然后向共享内存中写入字符串“world”。在两个进程完成之后,程序将删除共享内存段。
阅读全文
相关推荐



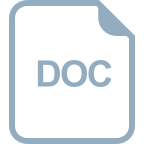
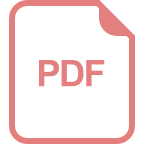
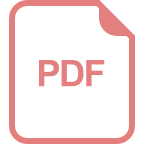
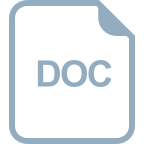
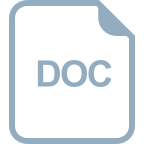




