使用队列来模拟停车场和便道上的车辆,每一次进出车辆都需要对队列进行操作。
时间: 2024-01-21 13:16:15 浏览: 37
好的,以下是一个基本的停车场和便道队列模拟程序:
```python
class ParkingLot:
def __init__(self, capacity):
self.capacity = capacity
self.parking_lot = []
self.waiting_queue = []
def park(self, car):
if len(self.parking_lot) < self.capacity:
self.parking_lot.append(car)
print(f"{car} has parked in the parking lot.")
else:
self.waiting_queue.append(car)
print(f"{car} is waiting in the queue.")
def leave(self, car):
if car in self.parking_lot:
self.parking_lot.remove(car)
print(f"{car} has left the parking lot.")
elif car in self.waiting_queue:
self.waiting_queue.remove(car)
print(f"{car} has left the waiting queue.")
else:
print(f"{car} is not found in the parking lot or the waiting queue.")
def status(self):
print(f"Parking lot capacity: {self.capacity}")
print(f"Number of cars parked: {len(self.parking_lot)}")
print(f"Number of cars waiting in the queue: {len(self.waiting_queue)}")
def display(self):
print("Cars parked in the parking lot:")
print(self.parking_lot)
print("Cars waiting in the queue:")
print(self.waiting_queue)
```
这个程序使用了一个 `ParkingLot` 类来表示停车场,其属性包括停车场的容量、停在停车场上的车辆列表以及等待队列。类中定义了 `park`、`leave`、`status` 和 `display` 方法来模拟进出车辆、查询状态以及展示当前停车场和便道的车辆情况。
以下是一个简单的测试:
```python
parking_lot = ParkingLot(2)
parking_lot.status()
parking_lot.park("car1")
parking_lot.park("car2")
parking_lot.park("car3")
parking_lot.status()
parking_lot.leave("car2")
parking_lot.status()
parking_lot.display()
```
输出:
```
Parking lot capacity: 2
Number of cars parked: 0
Number of cars waiting in the queue: 0
car1 has parked in the parking lot.
car2 has parked in the parking lot.
car3 is waiting in the queue.
Parking lot capacity: 2
Number of cars parked: 2
Number of cars waiting in the queue: 1
car2 has left the parking lot.
Parking lot capacity: 2
Number of cars parked: 1
Number of cars waiting in the queue: 1
Cars parked in the parking lot:
['car1']
Cars waiting in the queue:
['car3']
```
在这个测试中,我们首先创建了一个停车场,容量为 2。接着我们查询了停车场的状态,此时停车场为空。然后我们依次让三辆车进入停车场,其中前两辆车可以直接停在停车场上,而第三辆车需要等待在便道上。接着我们让第二辆车离开了停车场,此时停车场中只有一辆车,便道上还有一辆车。最后我们展示了当前停车场和便道上的车辆情况。
相关推荐
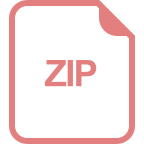
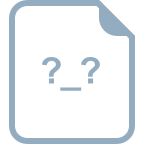















