private Integer changeAmount(List<OccupancyRateDTO> occupancyRates){ for (OccupancyRateDTO dto : occupancyRates) { if (dto.getAdult() == 2 && dto.getChild() == 0){ Number amount = (Number)dto.getAmountAfterTax(); } } return null; }我需要将dto.getAmountAfterTax的Double转换成Integer
时间: 2024-03-23 20:36:01 浏览: 131
您可以使用以下代码将 Double 转换为 Integer:
```
private Integer changeAmount(List<OccupancyRateDTO> occupancyRates){
for (OccupancyRateDTO dto : occupancyRates) {
if (dto.getAdult() == 2 && dto.getChild() == 0){
Double amountAfterTax = dto.getAmountAfterTax();
Integer amount = amountAfterTax.intValue();
dto.setAmountAfterTax(amount.doubleValue());
}
}
return null;
}
```
代码中,使用 `Double` 的 `intValue()` 方法将 `Double` 类型的 `amountAfterTax` 转换为 `Integer` 类型的 `amount`,然后再使用 `Double` 的 `doubleValue()` 方法将 `Integer` 类型的 `amount` 转换为 `Double` 类型,最终将转换后的 `amount` 赋值给 `dto` 的 `amountAfterTax` 属性。
相关问题
给你一个List<Module>的集合要封装成 private Integer id; private Integer pid; private String name; private String path; private String redirect; private String component; private String icon; private String key; private List<MoudleDto> children;这样的一个类,要是Module类中父id不是空,就要把找出list中的父id的Module对象要是这个对象没有父id了,并把之前的那个有父id的封装成它的的children,直到list中的Moudle封装完毕,这个逻辑用java代码该怎么做到
你可以使用递归的方式来封装这个 `List<Module>` 集合成为 `MoudleDto` 类的对象,按照父子关系构建 `children` 层级。以下是一个示例代码:
```java
public class Module {
private Integer id;
private Integer parentId;
private String name;
private String path;
// 其他属性省略
// 省略构造方法和其他 getter/setter 方法
}
public class MoudleDto {
private Integer id;
private Integer pid;
private String name;
private String path;
// 其他属性省略
private List<MoudleDto> children;
// 省略构造方法和其他 getter/setter 方法
}
public class ModuleUtil {
public static List<MoudleDto> buildModuleTree(List<Module> moduleList) {
Map<Integer, MoudleDto> moduleMap = new HashMap<>();
// 构建模块映射表
for (Module module : moduleList) {
MoudleDto dto = new MoudleDto();
dto.setId(module.getId());
dto.setPid(module.getParentId());
dto.setName(module.getName());
dto.setPath(module.getPath());
// 设置其他属性
moduleMap.put(module.getId(), dto);
}
// 构建模块树
List<MoudleDto> rootModules = new ArrayList<>();
for (MoudleDto moduleDto : moduleMap.values()) {
Integer parentId = moduleDto.getPid();
if (parentId == null) {
rootModules.add(moduleDto);
} else {
MoudleDto parentModule = moduleMap.get(parentId);
if (parentModule != null) {
parentModule.getChildren().add(moduleDto);
}
}
}
return rootModules;
}
}
```
在上述示例中,我们首先构建一个 `Map<Integer, MoudleDto>` 对象,用来存储每个模块的映射关系。然后,我们遍历 `moduleList` 集合,构建 `MoudleDto` 对象,并将其存储到映射表中。
接下来,我们遍历映射表,根据每个模块的父模块 ID,将其添加到父模块的 `children` 集合中。如果某个模块没有父模块,则将其添加到根模块集合中。
最后,我们返回根模块集合,即构建好的模块树。
请注意,在示例代码中,我假设 `Module` 类具有对应的属性和方法,并提供了相应的构造方法和 getter/setter 方法。你需要根据你的实际情况进行相应的调整和扩展。
你可以通过调用 `buildModuleTree` 方法并传入 `List<Module>` 对象来获取封装好的带有子模块的模块树。
Java List1<通货code,list<dto2> dto2<受渡日,残金额>,最后输出受渡日相同,通货相同的数据
好的,您的问题是关于Java的List和DTO的操作,您可以使用以下代码来实现您的需求:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
// 创建List和DTO对象
List<DTO1> list = new ArrayList<>();
DTO1 dto1_1 = new DTO1("code1", new DTO2("2022-01-01", 100));
DTO1 dto1_2 = new DTO1("code1", new DTO2("2022-01-02", 200));
DTO1 dto1_3 = new DTO1("code2", new DTO2("2022-02-01", 300));
DTO1 dto1_4 = new DTO1("code2", new DTO2("2022-02-02", 400));
list.add(dto1_1);
list.add(dto1_2);
list.add(dto1_3);
list.add(dto1_4);
// 创建Map对象,用于存储结果数据
Map<String, Map<String, List<Integer>>> resultMap = new HashMap<>();
// 遍历List对象
for (DTO1 dto1 : list) {
String code = dto1.getCode();
String date = dto1.getDto2().getDate();
int amount = dto1.getDto2().getAmount();
// 判断Map中是否已有该通货的数据
if (resultMap.containsKey(code)) {
Map<String, List<Integer>> innerMap = resultMap.get(code);
// 判断内部Map中是否已有该受渡日的数据
if (innerMap.containsKey(date)) {
List<Integer> amountList = innerMap.get(date);
amountList.add(amount);
} else {
List<Integer> amountList = new ArrayList<>();
amountList.add(amount);
innerMap.put(date, amountList);
}
} else {
Map<String, List<Integer>> innerMap = new HashMap<>();
List<Integer> amountList = new ArrayList<>();
amountList.add(amount);
innerMap.put(date, amountList);
resultMap.put(code, innerMap);
}
}
// 输出结果
for (String code : resultMap.keySet()) {
Map<String, List<Integer>> innerMap = resultMap.get(code);
for (String date : innerMap.keySet()) {
List<Integer> amountList = innerMap.get(date);
System.out.println("code: " + code + ", date: " + date + ", amount: " + amountList);
}
}
}
}
class DTO1 {
private String code;
private DTO2 dto2;
public DTO1(String code, DTO2 dto2) {
this.code = code;
this.dto2 = dto2;
}
public String getCode() {
return code;
}
public DTO2 getDto2() {
return dto2;
}
}
class DTO2 {
private String date;
private int amount;
public DTO2(String date, int amount) {
this.date = date;
this.amount = amount;
}
public String getDate() {
return date;
}
public int getAmount() {
return amount;
}
}
```
这段代码中,我们首先定义了两个DTO类,DTO1包含通货code和一个DTO2对象,DTO2包含受渡日和残金额。然后,我们在List中添加了一些DTO1对象,这些对象包含不同的通货code和不同的受渡日和残金额。
接下来,我们创建了一个Map对象,用于存储结果数据。在遍历List对象时,我们将每个DTO1对象中的数据逐一提取出来,并根据通货code和受渡日将其存储到Map对象中。最后,我们遍历Map对象,输出存储的结果数据。
需要注意的是,这段代码中的输出结果并没有按照受渡日相同、通货相同的顺序输出,如果您需要按照这个顺序输出,可以在遍历Map对象时进行一些排序操作。
阅读全文
相关推荐
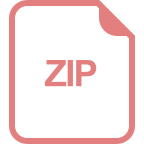
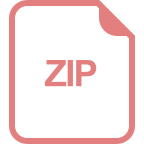
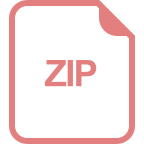












