设计一个判别表达式中左,右括号是否配对出现的算法,采用( )数据结构最佳
时间: 2023-04-08 15:02:52 浏览: 213
答案:可以使用栈来实现。遍历表达式,遇到左括号就将其压入栈中,遇到右括号就将栈顶元素弹出,如果弹出的元素不是与当前右括号匹配的左括号,则表达式中的左右括号不配对。最后,如果栈为空,则表达式中的左右括号全部配对。使用栈的时间复杂度为O(n),空间复杂度为O(n)。
相关问题
设计一个判别表达式中括号是否配对的算法,采用什么数据结构最佳
可以使用栈这种数据结构来实现括号匹配的算法。具体的实现过程如下:
1. 遍历表达式中的每一个字符,如果是左括号(包括小括号、中括号和大括号)则将其入栈。
2. 如果是右括号,取出栈顶元素进行匹配,如果匹配成功则弹出栈顶元素,否则表达式中括号不配对。
3. 遍历完整个表达式后,如果栈为空,则表达式中括号配对,否则表达式中括号不配对。
在这个算法中,栈的作用是记录左括号,在遇到右括号时进行匹配。因此使用栈这种数据结构最为合适。
以下是一个使用栈实现括号匹配的 Python 代码示例:
```python
def is_valid_expression(exp):
stack = []
for c in exp:
if c in ['(', '[', '{']:
stack.append(c)
elif c in [')', ']', '}']:
if not stack:
return False
top = stack.pop()
if (c == ')' and top != '(') or \
(c == ']' and top != '[') or \
(c == '}' and top != '{'):
return False
return not stack
```
该算法的时间复杂度是 O(n),其中 n 表示表达式的长度。
设计一个算法判别算术表达式中的圆括号是否配对正确
可以使用栈来判断算术表达式中的圆括号是否配对正确。
具体步骤如下:
1. 从左到右遍历表达式中的每个字符。
2. 如果遇到左括号,将其压入栈中。
3. 如果遇到右括号,判断栈顶元素是否为左括号。如果是,则将栈顶元素弹出;如果不是,则表达式中的圆括号不配对正确。
4. 遍历完整个表达式后,如果栈为空,则表达式中的圆括号配对正确;如果栈不为空,则表达式中的圆括号不配对正确。
例如,对于表达式 (1+2)*(3-4),可以按照上述步骤进行判断:
1. 遇到左括号 (,将其压入栈中。
2. 遇到数字 1、+、数字 2、),依次进行处理。
3. 遇到左括号 (,将其压入栈中。
4. 遇到数字 3、-、数字 4、),依次进行处理。
5. 遍历完整个表达式后,栈为空,表明表达式中的圆括号配对正确。
因此,可以设计一个基于栈的算法来判断算术表达式中的圆括号是否配对正确。
相关推荐
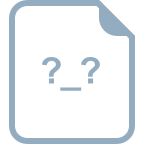






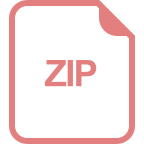
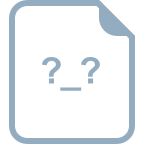
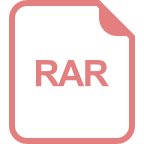
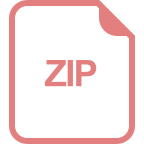